Native Mobile App Development: Mastering Android with Kotlin
Kotlin is the future of Android development. Learn why it’s time to make the switch!
Building a mobile app isn’t just about lines of code. It’s about creating experiences that resonate. Every tap, every swipe—crafted with precision.
When I first decided to get into Android development, the landscape was dominated by Java. Reliable, yes. But something was missing. Enter Kotlin. Suddenly, development felt different. Cleaner. More intuitive. It wasn’t just a new language; it was a new way of thinking.
Kotlin transformed the way we approached problems. Its concise syntax cut through the noise, letting us focus on what truly mattered—delivering value to users. The frustration of verbose code? Gone. In its place, a streamlined process that accelerated our development cycles without sacrificing quality.
But mastery doesn’t happen overnight. It requires dedication, understanding, and a willingness to embrace change. For early-stage founders dreaming of their first big launch, engineering managers seeking to optimize their teams, or senior executives aiming to drive technological excellence, Kotlin offers a powerful toolset to elevate your Android projects.
Imagine building apps that are not only robust and scalable but also a joy to develop. Apps that stand out in a crowded market, thanks to the efficiency and elegance Kotlin brings to the table. This journey isn’t just about adopting a new language; it’s about unlocking potential and pushing the boundaries of what’s possible.
Join me as we explore the depths of native Android development with Kotlin. Let’s uncover the strategies, best practices, and real-world applications that can turn your mobile vision into reality. Together, we’ll master Kotlin and shape the future of Android development.
The Evolution of Android Development
Android, since its inception, has been a dominant force in the mobile operating system market. Its open-source nature and extensive customization capabilities have made it a preferred choice for both developers and businesses aiming to reach a broad audience. The shift from Java to Kotlin as the preferred language for Android development marks a significant milestone in this evolution.
Why Kotlin?
Kotlin, introduced by JetBrains in 2011, has been officially supported by Google for Android development since 2017. Its concise syntax, null safety, and interoperability with Java make it an attractive choice for developers seeking to enhance productivity and reduce boilerplate code. For organizations, Kotlin represents not just a language but a strategic asset that can streamline development processes and improve app performance.
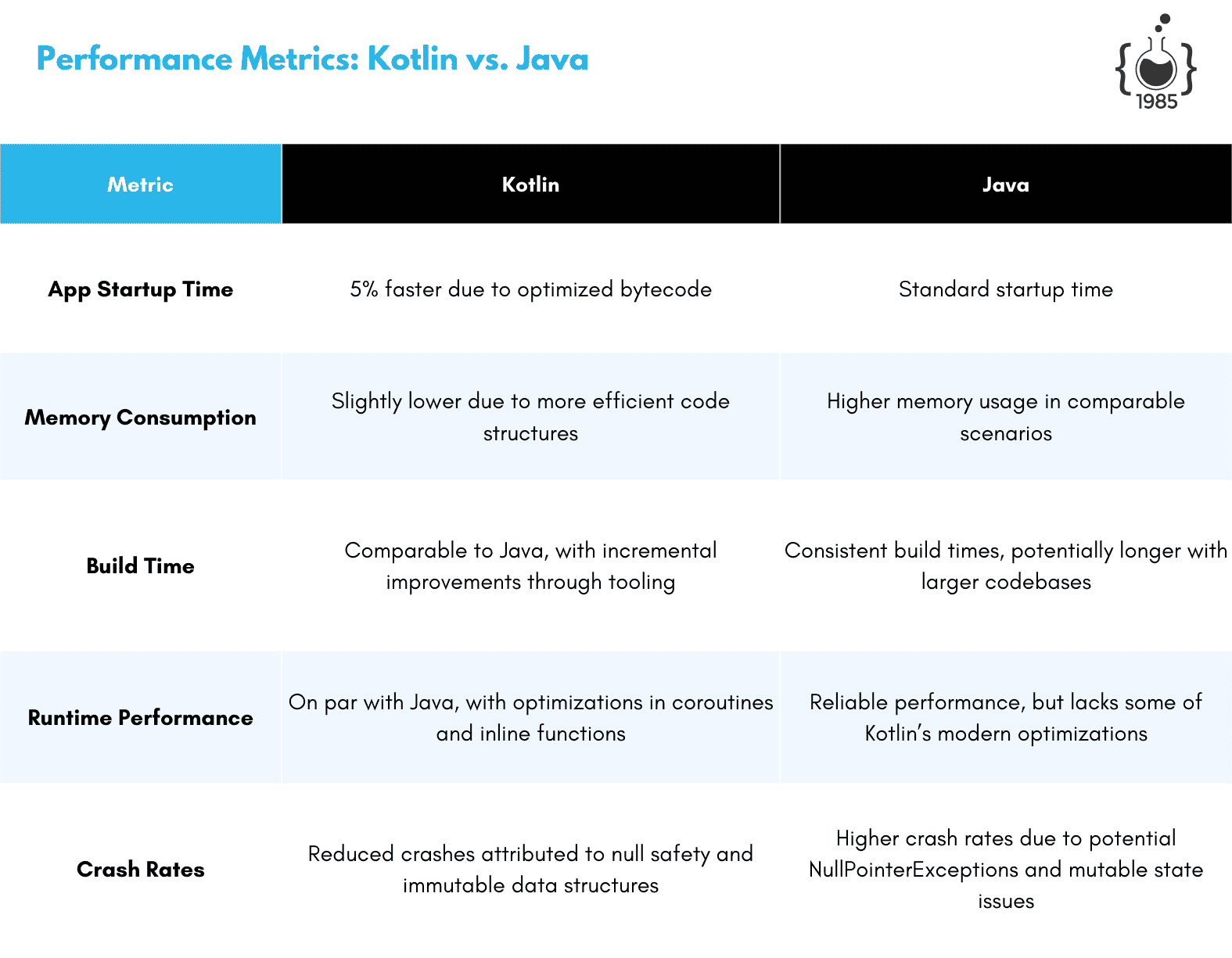
Advantages of Kotlin in Android Development
1. Conciseness and Readability
Kotlin's syntax is more concise than Java's, reducing the amount of boilerplate code required. This conciseness leads to increased readability and maintainability, which are crucial for large codebases and long-term projects.
Example:
Consider a simple Hello World
application.
Java:
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
}
Kotlin:
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
}
}
While both snippets are similar, Kotlin eliminates the need for semicolons and offers more streamlined syntax, which becomes increasingly beneficial as applications grow in complexity.
2. Null Safety
One of the most common sources of bugs in Java is the infamous NullPointerException
. Kotlin addresses this by incorporating null safety into its type system, distinguishing between nullable and non-nullable types.
Practical Implication:
In a large-scale application where multiple teams collaborate, Kotlin's null safety can significantly reduce runtime crashes, enhancing the app's reliability and user experience.
3. Interoperability with Java
Kotlin is fully interoperable with Java, allowing developers to gradually migrate existing Java codebases to Kotlin without disrupting ongoing projects. This interoperability facilitates a smoother transition and protects the investment in existing Java infrastructure.
4. Extension Functions
Kotlin's extension functions allow developers to extend existing classes with new functionality without inheriting from them. This feature promotes cleaner code and adheres to the Open/Closed Principle, a key tenet of software design.
Use Case:
Imagine enhancing the functionality of a third-party library without modifying its source code. Extension functions make this possible, enabling customization while maintaining the integrity of external dependencies.
Implementing Kotlin in Your Android Projects
Transitioning to Kotlin requires strategic planning and execution, especially for organizations with established Java codebases. Here are specific tactics to ensure a seamless adoption of Kotlin in Android development.
1. Incremental Migration
Rather than overhauling the entire codebase at once, adopt an incremental approach. Start by introducing Kotlin in new modules or features while maintaining existing Java code. This strategy minimizes disruption and allows teams to familiarize themselves with Kotlin's nuances gradually.
Example Table: Incremental Migration Plan
Phase | Action | Expected Outcome |
---|---|---|
Assessment | Audit existing codebase for Kotlin integration | Identify modules suitable for migration |
Pilot Project | Implement a small feature in Kotlin | Gain hands-on experience with minimal risk |
Gradual Integration | Migrate specific modules incrementally | Ensure stability and maintain code quality |
Full Adoption | Transition remaining Java code to Kotlin | Achieve a unified Kotlin-based codebase |
2. Training and Skill Development
Investing in training ensures that your development team is proficient in Kotlin. Organize workshops, provide access to online courses, and encourage participation in Kotlin communities. A well-trained team can reach Kotlin's full potential, driving innovation and efficiency.
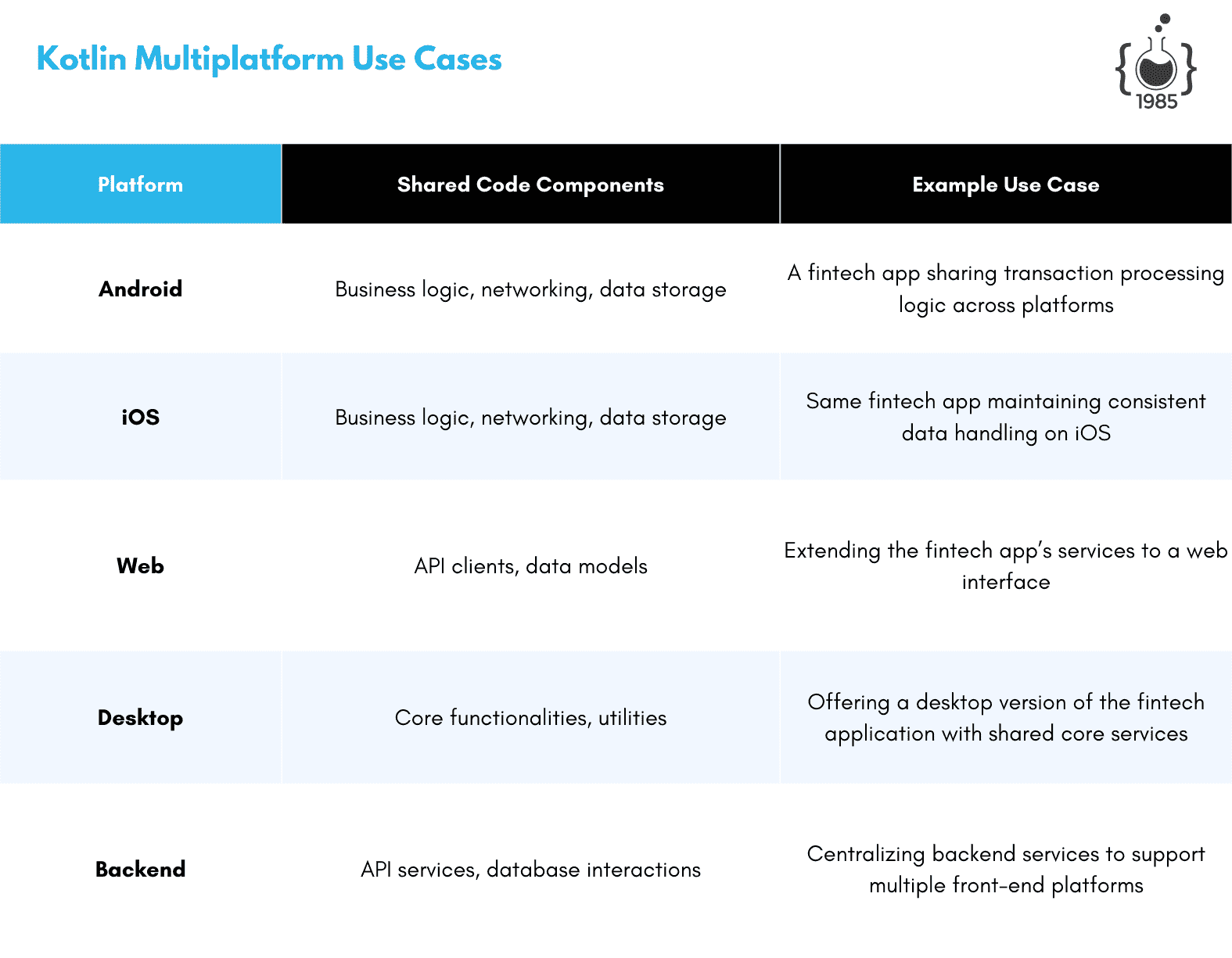
3. Work with Kotlin Multiplatform
Kotlin Multiplatform allows for code sharing across different platforms, including Android, iOS, and web. For organizations aiming to develop cross-platform applications, this feature can lead to significant time and resource savings.
Practical Example:
A fintech startup developing a mobile app for both Android and iOS can use Kotlin Multiplatform to share business logic, reducing duplication and ensuring consistency across platforms.
4. Adopt Modern Android Development Practices
Embrace Android's modern development practices, such as Jetpack Compose for UI development, alongside Kotlin. Combining these tools fosters a more cohesive and efficient development environment.
Use Case:
Integrating Jetpack Compose with Kotlin allows for declarative UI development, making it easier to manage complex user interfaces and enhancing collaboration between designers and developers.
Case Studies: Kotlin in Action
Understanding how Kotlin has been successfully implemented in real-world scenarios provides valuable insights into its practical benefits and challenges.
1. Airbnb's Transition to Kotlin
Airbnb, a global leader in the travel industry, embarked on transitioning its Android codebase from Java to Kotlin. The decision was driven by the need for increased developer productivity and code safety.
Outcomes:
- Reduced Codebase Size: Kotlin's conciseness led to a significant reduction in the overall codebase, simplifying maintenance.
- Enhanced Safety: Null safety features decreased runtime crashes, improving the app's reliability.
- Developer Satisfaction: Increased job satisfaction among developers due to Kotlin's modern features and reduced boilerplate code.
Key Takeaway:
A phased migration, coupled with comprehensive training, can lead to substantial improvements in code quality and developer morale.
2. Trello's Adoption of Kotlin
Trello, a project management tool used by millions, integrated Kotlin into its Android application to enhance performance and streamline development workflows.
Outcomes:
- Improved Performance: Kotlin's efficient handling of asynchronous tasks contributed to smoother app performance.
- Seamless Interoperability: Maintaining interoperability with existing Java modules ensured that ongoing projects were not disrupted.
- Scalability: Kotlin's features facilitated the scalability of Trello's app, accommodating a growing user base and feature set.
Key Takeaway:
Kotlin's interoperability and performance optimizations make it an ideal choice for applications requiring high scalability and efficiency.
Best Practices for Mastering Kotlin in Android Development
To fully utilize the full potential of Kotlin, adhering to best practices is essential. These practices not only enhance code quality but also ensure maintainability and scalability.
1. Embrace Functional Programming Paradigms
Kotlin supports functional programming features such as lambda expressions, higher-order functions, and immutability. Applying these paradigms can lead to more predictable and testable code.
Practical Example:
Using higher-order functions to handle asynchronous operations can simplify code and reduce the likelihood of callback hell.
fun fetchData(callback: (Result) -> Unit) {
// Asynchronous operation
}
2. Utilize Coroutines for Asynchronous Programming
Kotlin Coroutines provide a robust framework for managing asynchronous tasks, replacing traditional callbacks with a more readable and maintainable approach.
Use Case:
In an application that requires fetching data from a remote server, coroutines can manage network requests efficiently without blocking the main thread.
suspend fun fetchData(): Data {
return withContext(Dispatchers.IO) {
// Network request
}
}
3. Implement Dependency Injection
Adopting dependency injection frameworks like Koin or Dagger enhances modularity and testability. It allows for better management of dependencies, leading to cleaner and more maintainable code.
Practical Example:
Using Koin for dependency injection can simplify the provisioning of services and repositories within the app, reducing tight coupling between components.
val appModule = module {
single { NetworkService() }
factory { Repository(get()) }
}
4. Adopt MVVM Architecture
Model-View-ViewModel (MVVM) is a widely recommended architectural pattern for Android development. It promotes a clear separation of concerns, making the codebase more manageable and scalable.
Use Case:
Implementing MVVM in a complex application allows different teams to work on the UI and business logic independently, streamlining the development process.
5. Write Unit and UI Tests
Ensuring code reliability through comprehensive testing is non-negotiable. Kotlin's features facilitate writing clear and concise tests, enhancing the overall quality of the application.
Practical Example:
Using frameworks like JUnit for unit testing and Espresso for UI testing ensures that individual components and the user interface function as intended.
@Test
fun testFetchData() {
val result = fetchData()
assertNotNull(result)
}
Overcoming Challenges in Kotlin Adoption
While Kotlin offers numerous advantages, transitioning to it can present challenges. Addressing these proactively ensures a smooth adoption process.
1. Learning Curve
For teams accustomed to Java, transitioning to Kotlin requires time and effort. Mitigate this by providing structured training programs and encouraging pair programming sessions to foster knowledge sharing.
2. Tooling and Integration
Ensuring that existing tools and frameworks are compatible with Kotlin is crucial. Most modern development tools support Kotlin, but thorough testing during migration can identify and resolve integration issues early.
3. Performance Optimization
While Kotlin generally performs on par with Java, certain features like inline functions and coroutines require careful optimization to avoid performance bottlenecks. Profiling and benchmarking during development can help identify and address these issues.
The Strategic Advantage of Kotlin in Enterprise Development
For mid-sized to large corporations, the strategic adoption of Kotlin can lead to significant competitive advantages.
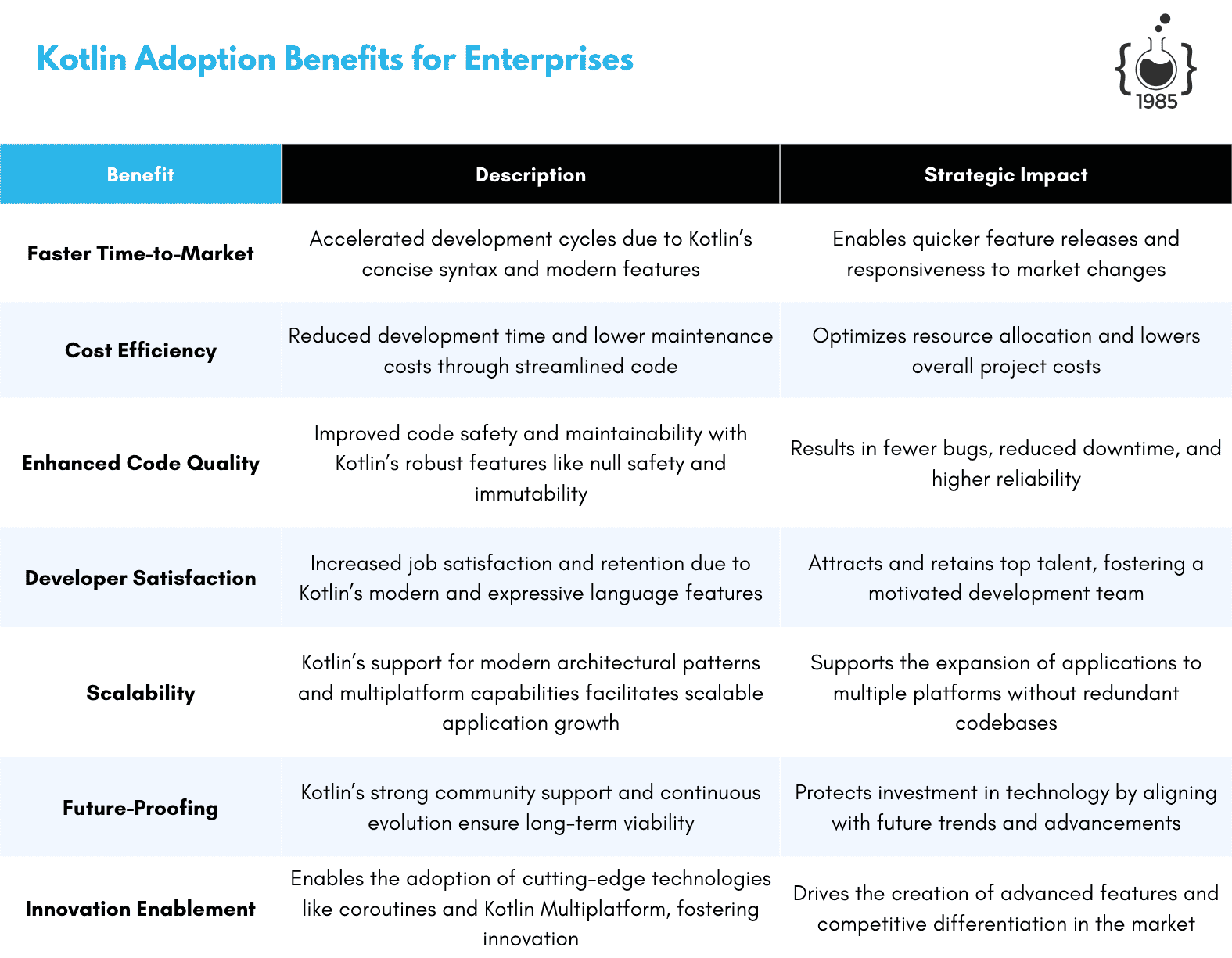
1. Faster Time-to-Market
Kotlin's concise syntax and modern features accelerate development cycles, enabling faster delivery of new features and updates. This agility is crucial in responding to market demands and staying ahead of competitors.
2. Enhanced Developer Productivity
By reducing boilerplate code and simplifying complex tasks, Kotlin allows developers to focus on delivering value rather than managing mundane coding details. This boost in productivity translates to cost savings and more efficient resource allocation.
3. Improved Code Quality and Maintainability
Kotlin's emphasis on safety and clarity results in cleaner, more maintainable codebases. For large organizations with extensive code repositories, this means easier onboarding of new developers and smoother collaboration across teams.
4. Future-Proofing Applications
As the mobile ecosystem continues to evolve, Kotlin's growing community and support ensure that applications built with it remain relevant and adaptable to future technological advancements.
Practical Steps for Decision-Makers
For executives and decision-makers contemplating the shift to Kotlin, a structured approach is essential to maximize benefits and minimize risks.
1. Assess Current Infrastructure
Evaluate the existing codebase, development workflows, and team expertise to determine the feasibility of integrating Kotlin. Identify areas where Kotlin can provide immediate benefits without disrupting ongoing projects.
2. Develop a Migration Roadmap
Create a detailed migration plan that outlines phases, milestones, and resource allocations. Prioritize modules that can quickly showcase Kotlin's advantages, building momentum for broader adoption.
3. Invest in Training and Development
Allocate resources for training programs that equip your development team with the necessary Kotlin skills. Encourage continuous learning through workshops, online courses, and participation in Kotlin communities.
4. Foster a Collaborative Culture
Promote collaboration between teams by establishing best practices and coding standards for Kotlin. Encourage knowledge sharing and mentorship to ensure consistent and high-quality code across the organization.
5. Monitor and Iterate
Continuously monitor the progress of Kotlin integration, gathering feedback from developers and stakeholders. Use this feedback to iterate on the migration strategy, addressing challenges and taking advantage of opportunities for improvement.
Future Trends in Kotlin and Android Development
Staying abreast of emerging trends ensures that your applications remain cutting-edge and competitive. Here are some anticipated developments in Kotlin and Android development.
1. Kotlin Multiplatform Expansion
Kotlin Multiplatform is expected to gain more traction, enabling even greater code sharing across diverse platforms. This trend will facilitate the development of unified applications, reducing duplication and enhancing consistency.
2. Integration with Emerging Technologies
As technologies like augmented reality (AR), virtual reality (VR), and machine learning (ML) become more prevalent, Kotlin's interoperability and flexibility position it well to integrate with these advancements, enabling the creation of innovative and immersive applications.
3. Enhanced Tooling and Frameworks
The Kotlin ecosystem is continuously evolving, with new tools and frameworks emerging to simplify development processes. Anticipate more sophisticated IDE integrations, debugging tools, and performance optimization utilities tailored for Kotlin.
4. Increased Emphasis on Security
With the growing importance of cybersecurity, Kotlin's features like null safety and immutability contribute to building more secure applications. Future developments may further enhance Kotlin's capabilities in this domain, providing developers with robust tools to safeguard their apps.
Conclusion
Mastering native Android development with Kotlin is not merely a technical endeavor but a strategic initiative that can propel organizations toward greater efficiency, innovation, and competitiveness. By embracing Kotlin's modern features, fostering a culture of continuous learning, and implementing structured migration strategies, businesses can realize the full potential of Android development.
For early-stage founders, engineering managers, senior executives, and decision-makers at mid-sized to large corporations, the journey to mastering Kotlin involves not only adopting a new programming language but also reimagining development workflows to align with contemporary best practices. The investment in Kotlin today paves the way for scalable, maintainable, and high-performing applications that meet the dynamic demands of the modern mobile landscape.
As the mobile ecosystem continues to evolve, those who strategically take advantage of Kotlin will be well-positioned to lead the charge in delivering exceptional user experiences and driving technological innovation.
References
- JetBrains Kotlin Documentation: https://kotlinlang.org/docs/home.html
- Google Android Developer Guides: https://developer.android.com/kotlin
- Airbnb Engineering Blog on Kotlin: https://medium.com/airbnb-engineering
Further Reading
- "Kotlin in Action" by Dmitry Jemerov and Svetlana Isakova
- "Android Programming with Kotlin for Beginners" by John Horton
- "Effective Kotlin" by Marcin Moskala