iOS App Architecture: MVVM vs MVC
Choosing between MVC and MVVM for your next iOS app? Explore the benefits, pitfalls, and practical use cases for each.
Choosing the Right Approach for Your Next iOS Project
If you’re developing iOS apps, you’re familiar with that nagging moment of choice—the one where you decide which architecture to use. The architecture of an app might not be the sexiest part of building software, but it's absolutely one of the most important. Because that moment is like deciding whether to build a house on sand or on bedrock. MVC, MVVM, or some other architectural pattern—the decision lays the foundation for everything that comes next.
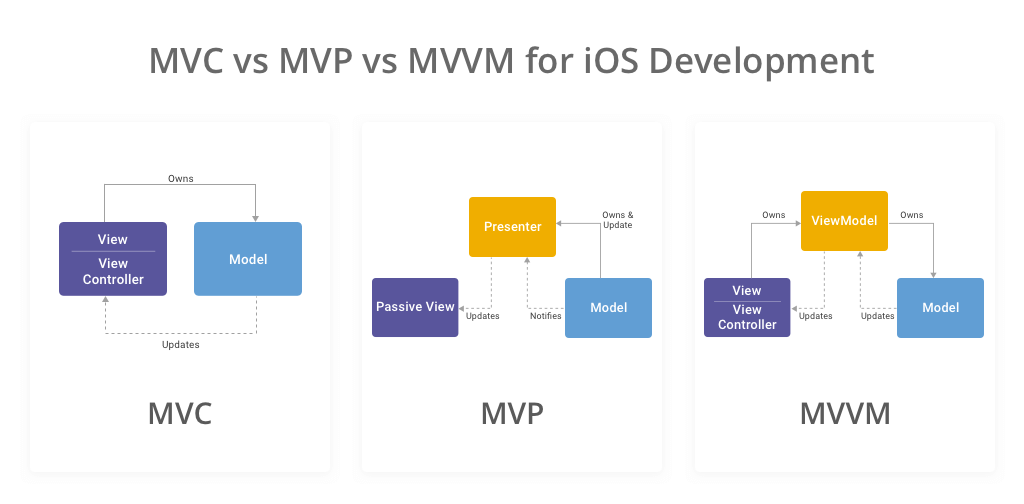
Let’s face it, choosing between MVC (Model-View-Controller) and MVVM (Model-View-ViewModel) can feel like choosing between two flavors of black coffee. Each has its own bite. Each offers a slightly different experience. And, ultimately, what you choose has less to do with one being “right” or “wrong”, but more with which best fits your workflow, team, and specific project quirks.
In this post, we're not diving into introductory material—let's skip the fluff—instead, we’re focusing on nuanced, industry-proven insights. As someone running an outsourced dev company, I’ve seen first-hand how choosing one pattern over the other can significantly influence maintenance costs, feature scalability, and even developer sanity. We'll look at real-world trade-offs, and by the end, you'll be able to see a clearer picture of which one might be your best pick for the next project.
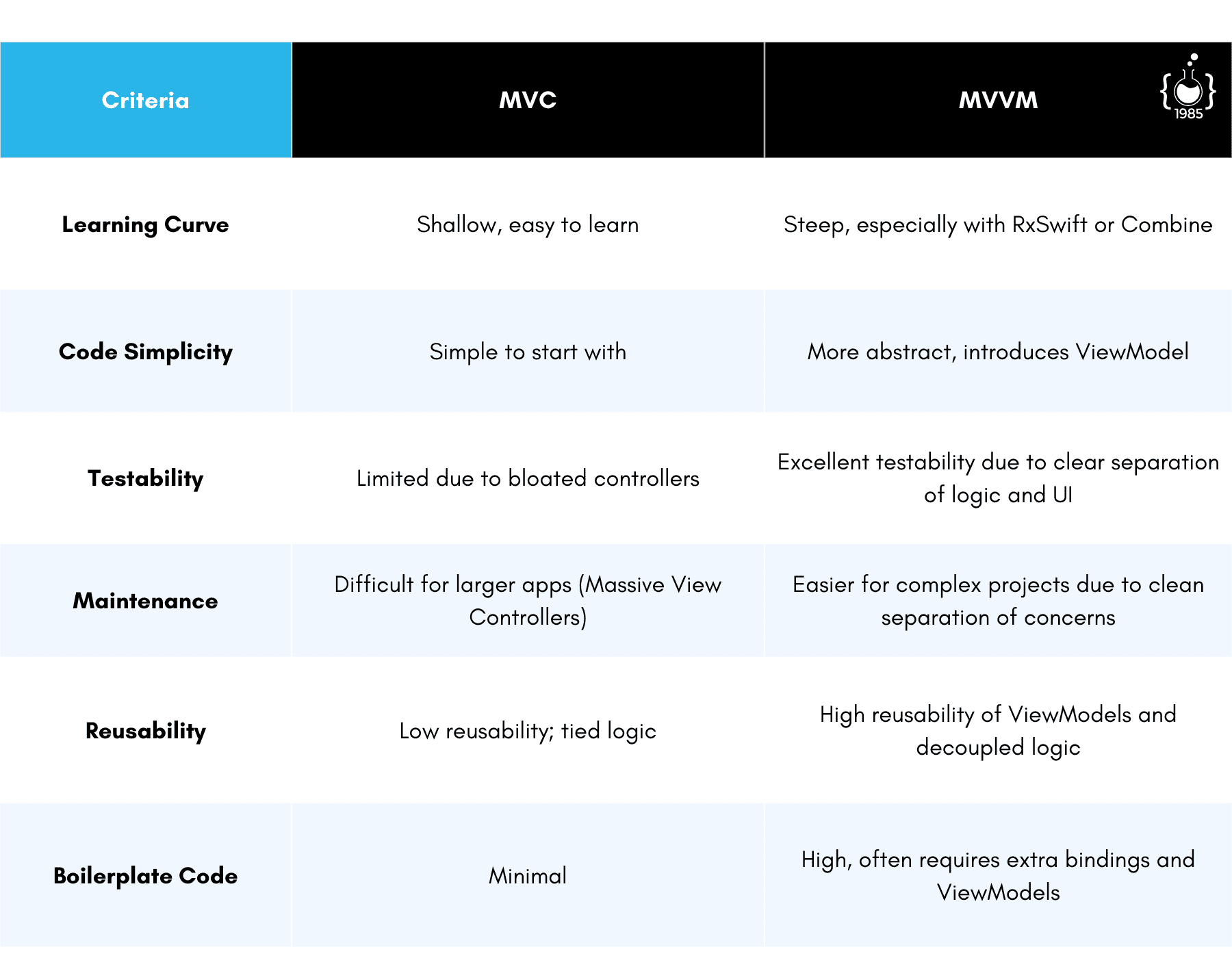
MVC: The "Classic" Approach
MVC has been the default choice for iOS developers since the early days of the App Store. And for good reason—it’s easy to pick up, well-documented, and the framework itself guides you towards it. Apple loves MVC. The UIKit, from its core, was designed with MVC in mind.
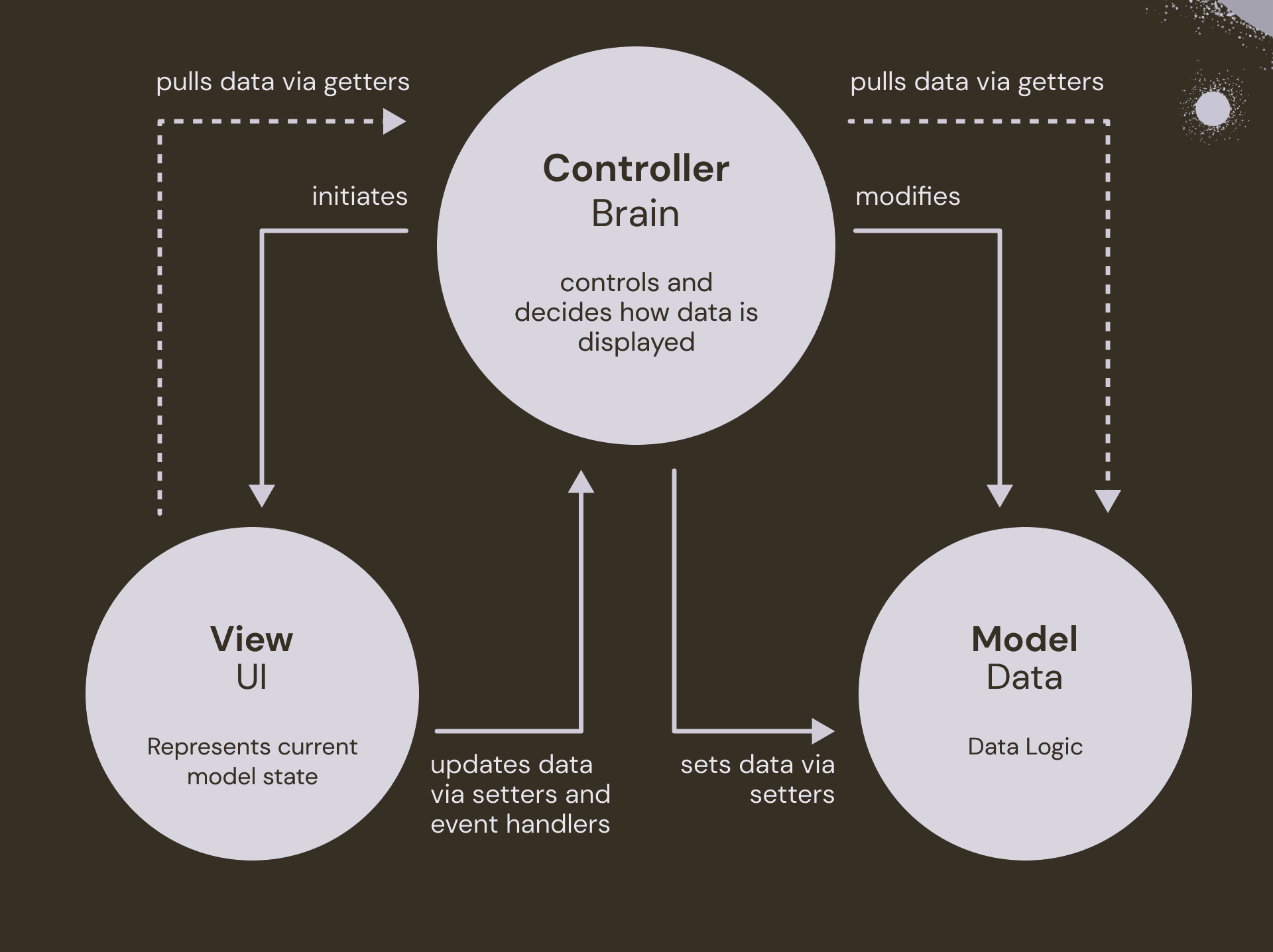
Benefits of MVC
MVC’s simplicity is its superpower. Here’s why:
Natural Segmentation: Splitting code into Models, Views, and Controllers comes with a natural appeal. You get a neat segmentation of roles: data in the Model, presentation in the View, and logic in the Controller. This helps teams new to the platform—especially if you’re ramping up junior developers or freelancers who need a minimal learning curve.
Straightforward Communication Flow: MVC is like a single highway—all communication flows in a predictable manner. Models update Controllers, Controllers update Views, and things are as simple as it sounds (at least at first). A junior dev can step into an MVC project and intuitively understand the data flow. The direct relationship means debugging is often simple, and the separation is tangible, which makes architectural refactoring (when it's called for) less intimidating.
Drawbacks of MVC
However, as anyone who’s built a real-world iOS app knows, MVC isn’t without its problems—particularly when your app starts to grow. Here’s where it starts to hurt:
Massive View Controller (MVC, ironically): Ah yes, the dreaded Massive View Controller problem. The pattern is so easy to understand, and so intuitive that developers inevitably end up placing too much responsibility in their Controllers. In many apps, View Controllers become bloated beasts, taking on everything from networking to data transformation. This results in controllers that can easily grow to 1000+ lines, becoming a bottleneck for team velocity and a playground for bugs.
Limited Testability: Unit testing in MVC is no picnic. The heavy logic sitting in View Controllers can be tricky to mock or isolate. Every developer loves a stable codebase, and with MVC, the more functionality you add, the harder that stability becomes to maintain—especially if you don’t have tests to back up your changes.
MVVM: The "New Cool Kid"
MVVM emerged as an alternative to MVC—something to help reduce the bloat and introduce better scalability. Pioneered by Microsoft for WPF applications, it’s a pattern that has resonated well in the world of iOS too, especially since reactive programming has become popular.
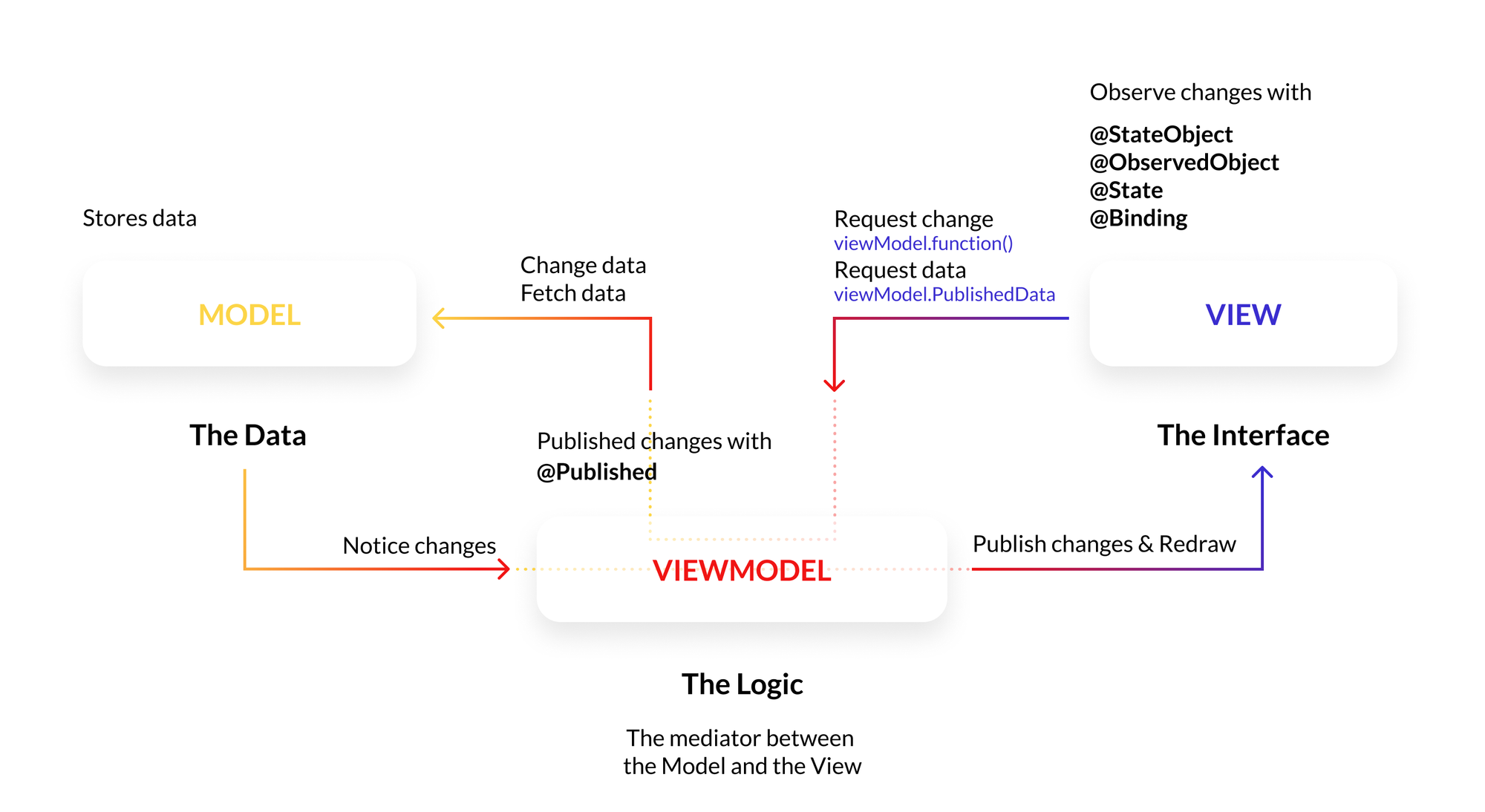
Benefits of MVVM
Improved Testability: MVVM pulls logic away from View Controllers and places it into ViewModels. This makes the app more modular, and this modularity leads to a codebase that's easier to test. Your ViewModel holds the logic, your View holds the UI, and the two talk through well-defined bindings—preferably through a reactive framework like RxSwift or Combine. Tests become simpler because you can unit test the ViewModel without worrying about the UI.
Better Reusability and Maintainability: The key point about MVVM is the separation of concerns. Since the ViewModel is independent of the View, you can easily tweak, upgrade, or replace the UI without affecting the business logic. Reusability in MVVM is excellent, especially when you work on feature-rich applications with multiple similar-looking components. Views become dumb—their only job is to reflect what the ViewModel tells them to.
Drawbacks of MVVM
Steep Learning Curve: MVVM is conceptually more complex than MVC. Introducing reactive bindings between the View and ViewModel can feel like overkill—especially for simpler applications or small teams who are trying to move fast. MVVM almost always benefits from a reactive framework, but frameworks like RxSwift introduce their own syntax and paradigms that take time to master.
Boilerplate Code: With MVVM, you’re introducing a new layer: the ViewModel. While this helps decouple logic, it also means a lot of extra code. Depending on the project, the sheer amount of code required to handle all the bindings and events can make development feel slower and cumbersome—especially in cases where a simple MVC Controller/View pairing would do just fine.
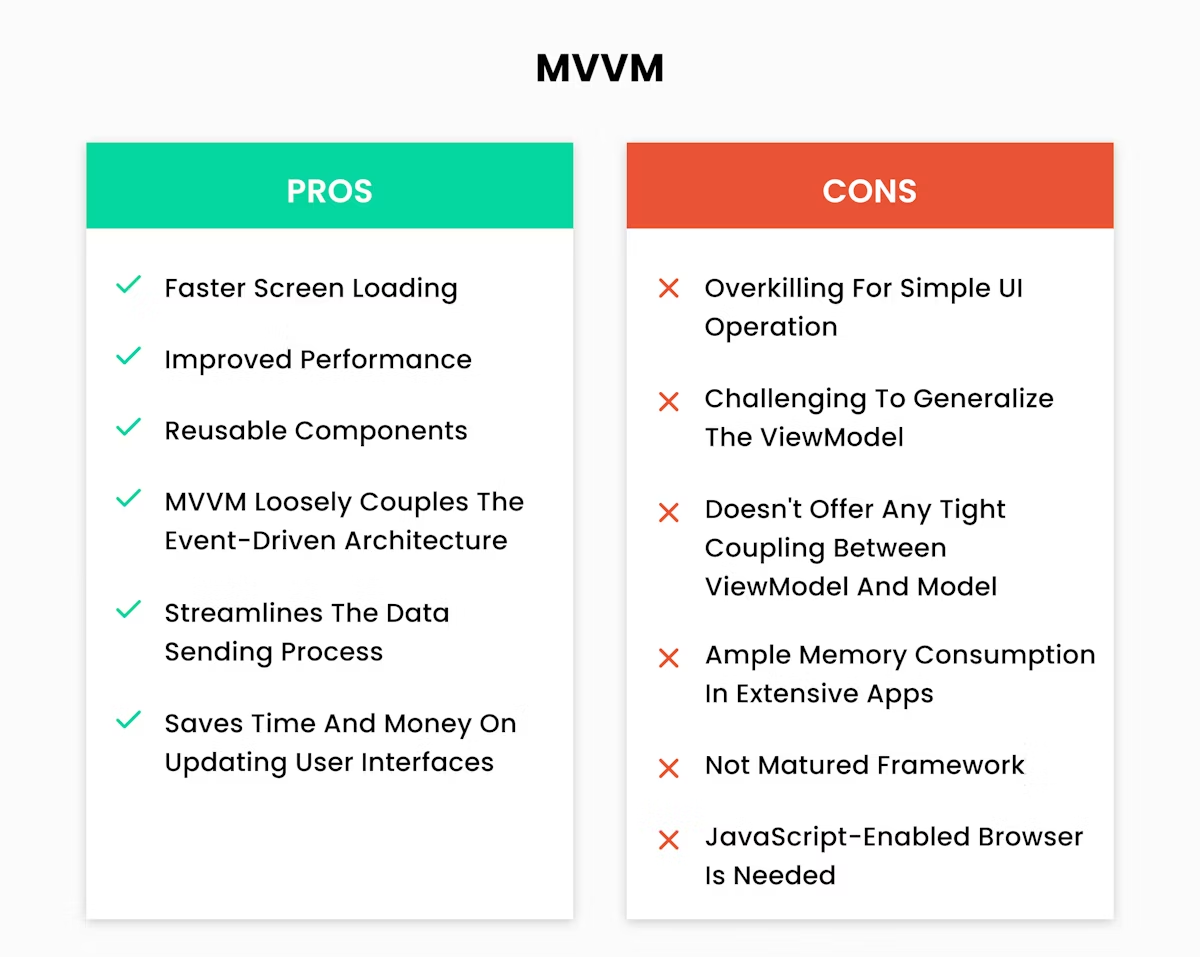
Real-World Considerations: Picking Between MVC and MVVM
Choosing the right pattern for an iOS app is seldom a black-and-white decision. Let’s walk through some considerations.
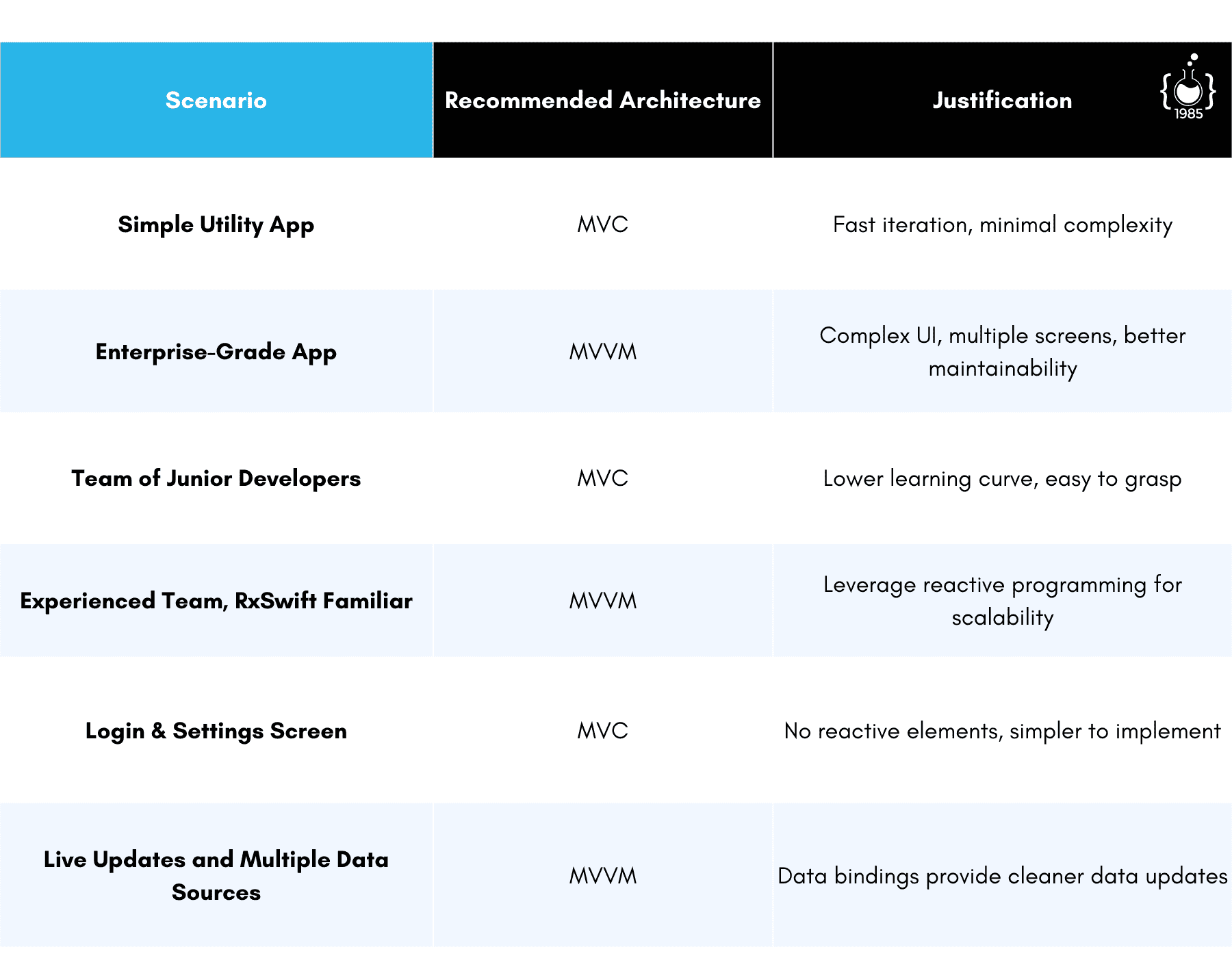
Team Skill Set
Your team’s experience with architectural patterns should be a key deciding factor. In our experience at 1985, when working with teams that are less experienced or ramping up quickly, we’ve found that sticking with MVC can be a lifesaver. Why? Because the simplicity of MVC aligns perfectly with developers still learning the ropes of app architecture.
On the other hand, MVVM works well with teams familiar with reactive programming and larger-scale projects. If your developers have solid experience with RxSwift, Combine, or other reactive frameworks, the benefits of MVVM become much more pronounced.
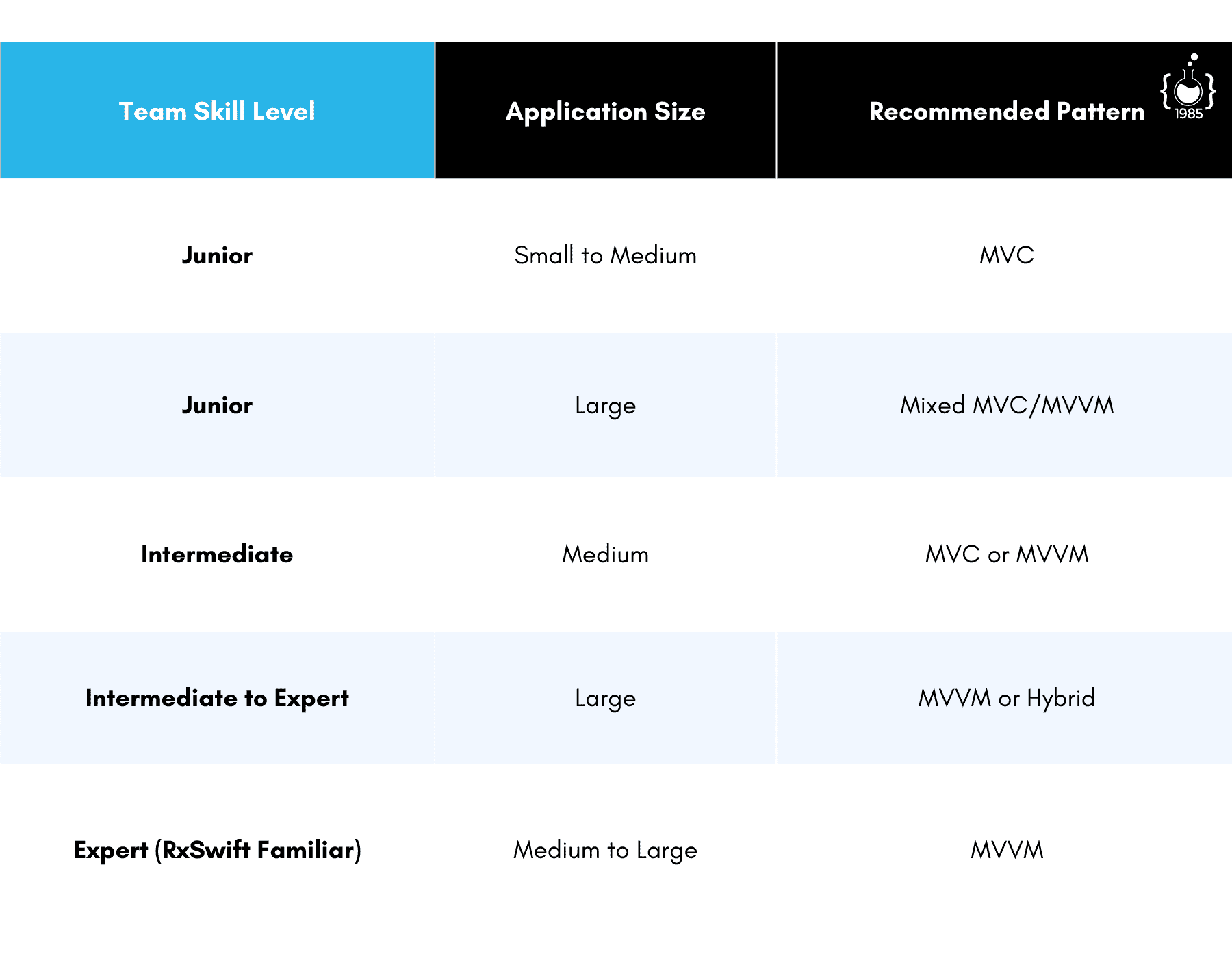
Application Size and Complexity
The size and complexity of your app also influence which architecture is ideal. A small utility app? MVC is the right choice. It’s simple, fast, and easy to iterate. But for applications that require scalability, complex data handling, or advanced UI requirements, MVVM often shines. Especially if you’re dealing with multiple screens where data is interdependent—the binding provided by MVVM can reduce a lot of headaches.
Take for example a recent project we tackled at 1985. It was an enterprise-grade app—hundreds of screens, a dynamic UI, and multiple data points syncing in real-time. We initially started with MVC, but after our ViewControllers became too bloated to manage, we pivoted to MVVM. The refactor cost us some time, but the payoff was worth it—the decoupling significantly reduced our maintenance overhead.
Third-Party Integrations and Reactive Frameworks
MVVM is particularly well-suited for apps using reactive frameworks. Bindings can feel magical—the ViewModel updates, and the View automatically changes without manual intervention. If your app needs to pull dynamic data from APIs or process heavy user input, this type of synchronization can create a seamless user experience.
However, do keep in mind that using frameworks like RxSwift or Combine is a skill unto itself. Reactive programming is powerful, but it introduces a whole new set of debugging challenges—often making bugs more subtle and harder to track. If your team isn’t familiar, a misconfigured reactive component can create spaghetti code that defeats MVVM’s purpose.
Mixed Architectures: The Reality of Modern iOS Development
Here’s something we’ve found often overlooked: rarely does an app’s architecture fit neatly into a single pattern. Modern iOS apps, especially at scale, often blend MVC, MVVM, and even other patterns like MVP (Model-View-Presenter). Different parts of an application have different needs, and sometimes using mixed architectures is the most pragmatic choice.
When to Mix
Imagine you’re building a social media application. For parts of the app like login screens or settings, where the logic is relatively simple and doesn’t require reactive components, MVC might suffice perfectly well. In contrast, for feeds with multiple data sources, live updates, and user interaction, MVVM paired with a reactive framework could provide a cleaner and more maintainable solution.
At 1985, we take a hybrid approach more often than not. We use MVC to get our core screens up and running quickly, which allows us to provide early builds to stakeholders and iterate. As complexity grows, or as screens start to require intricate user interactions, we gradually introduce MVVM—segmenting ViewModels from controllers.
Another trick is to keep the core business logic completely separate in a framework or service layer that is used by both MVC and MVVM parts. This way, even if you blend patterns, your core logic remains untouched and easily testable.
Choosing Wisely
No single pattern is a magic bullet—which is probably what makes architectural decisions so interesting (and frustrating) at times. MVC, with its simplicity, is great for getting started and suits less complex projects or junior teams. But MVVM, though more complex, provides the decoupling and testability required for scalable and maintainable applications.
Consider the project scope, the team's skill level, the complexity of the application, and your end goals. MVC can get you started quickly, while MVVM will provide stability and testability in the long run. Don’t be afraid to blend the two where it makes sense.
At 1985, our goal is always the same: ship high-quality software that we can maintain and grow over time. Whether it’s MVC, MVVM, or a hybrid, the real key is to stay adaptable—to evolve with your app’s changing requirements and the growing skillset of your team. The architecture isn’t just a technical decision; it’s a strategic one that influences team velocity, happiness, and ultimately, the user experience.