Clean Up Your API Spaghetti
Simplify your API structure with expert tips to improve developer productivity and system scalability.
APIs are the backbone of modern software ecosystems. They connect, enable, and empower systems to communicate seamlessly—at least, in theory. But let’s be honest: most of us have been there. What starts as a clean, well-organized API structure can quickly morph into a tangled mess. A spaghetti of endpoints, poorly documented parameters, redundant methods, and inconsistent error handling. Sound familiar?
Cleaning up a messy API architecture isn’t just a technical exercise. It’s about restoring sanity, improving developer productivity, and creating systems that scale. Let’s dive into actionable strategies to simplify complex API architectures and implement cleaner integration patterns. Whether you’re a software developer, architect, or CTO, these strategies can make a real difference.
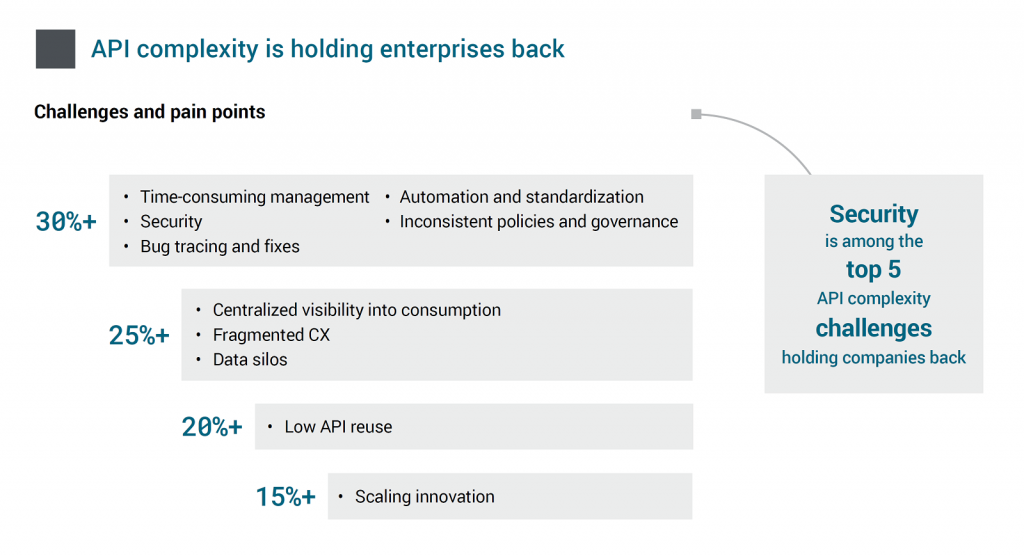
Why APIs Get Messy
APIs rarely become tangled overnight. The messiness often builds up over time, fueled by real-world challenges:
Rapid Growth:
When startups scale fast, APIs evolve in reaction to immediate needs. This "just make it work" mindset leads to shortcuts that eventually snowball. For example, an initial simple endpoint like /getUser
might evolve into multiple similar endpoints like /getUserDetails
, /getUserProfile
, and /getUserSettings
, each with slight variations that create redundancy and confusion.
Multiple Contributors:
Large teams with different coding styles and priorities introduce inconsistencies. One developer’s elegant solution can clash with another’s quick fix. Without a unified approach, APIs can end up with mismatched naming conventions, varied authentication methods, and inconsistent response formats, making maintenance a nightmare.
Legacy Systems:
Integrating with outdated systems often means carrying forward quirks and inefficiencies. Legacy APIs might lack proper documentation, use outdated protocols, or have limited functionality, forcing developers to implement workarounds that complicate the overall architecture.
Lack of Standards:
Without clear guidelines, APIs end up with mismatched naming conventions, undocumented endpoints, and inconsistent response formats. This lack of standardization can lead to confusion among developers, increased onboarding time, and a higher likelihood of errors.
A messy API isn’t just an eyesore. It’s a productivity killer. Developers waste time debugging, clients experience more errors, and every change becomes risky. Moreover, a tangled API can hinder the adoption of new technologies and integrations, limiting the potential growth and adaptability of your software ecosystem.
Step 1: Audit Your API Landscape
The first step to untangling API spaghetti is understanding the problem. Conduct a thorough audit of your API ecosystem. Look at:
Endpoint Redundancy:
Are there multiple endpoints performing similar tasks? For instance, having both /createUser
and /registerUser
might serve the same purpose but create confusion. Consolidating these into a single, well-defined endpoint can streamline operations and reduce maintenance overhead.
Documentation Gaps:
How well are the APIs documented? Can someone new to the team get up to speed without handholding? Comprehensive documentation should include clear descriptions of each endpoint, parameters, response formats, and example use cases. Tools like Swagger or Postman can help visualize and document APIs effectively.
Response Consistency:
Do all endpoints follow the same structure for success and error responses? Inconsistent responses can lead to integration issues and increase the complexity of error handling. Establishing a standardized response format ensures predictability and simplifies client-side processing.
Error Handling:
Is error handling uniform, or do different endpoints behave unpredictably? Consistent error codes and messages help developers quickly identify and resolve issues. Establish a clear error-handling strategy that outlines common error codes, their meanings, and recommended actions.
Tools to Help:
Use tools like Swagger or Postman to visualize your APIs. These platforms help identify gaps and inconsistencies at a glance. For larger ecosystems, automated linting tools like Spectral can enforce standards and highlight discrepancies. Additionally, API management platforms like Apigee or AWS API Gateway offer features for monitoring, versioning, and securing your APIs.

Pro Tip:
Audit logs can reveal which endpoints are most frequently used. Focus your cleanup efforts on high-traffic areas first for maximum impact. Prioritizing these areas can enhance performance and user satisfaction where it matters most.
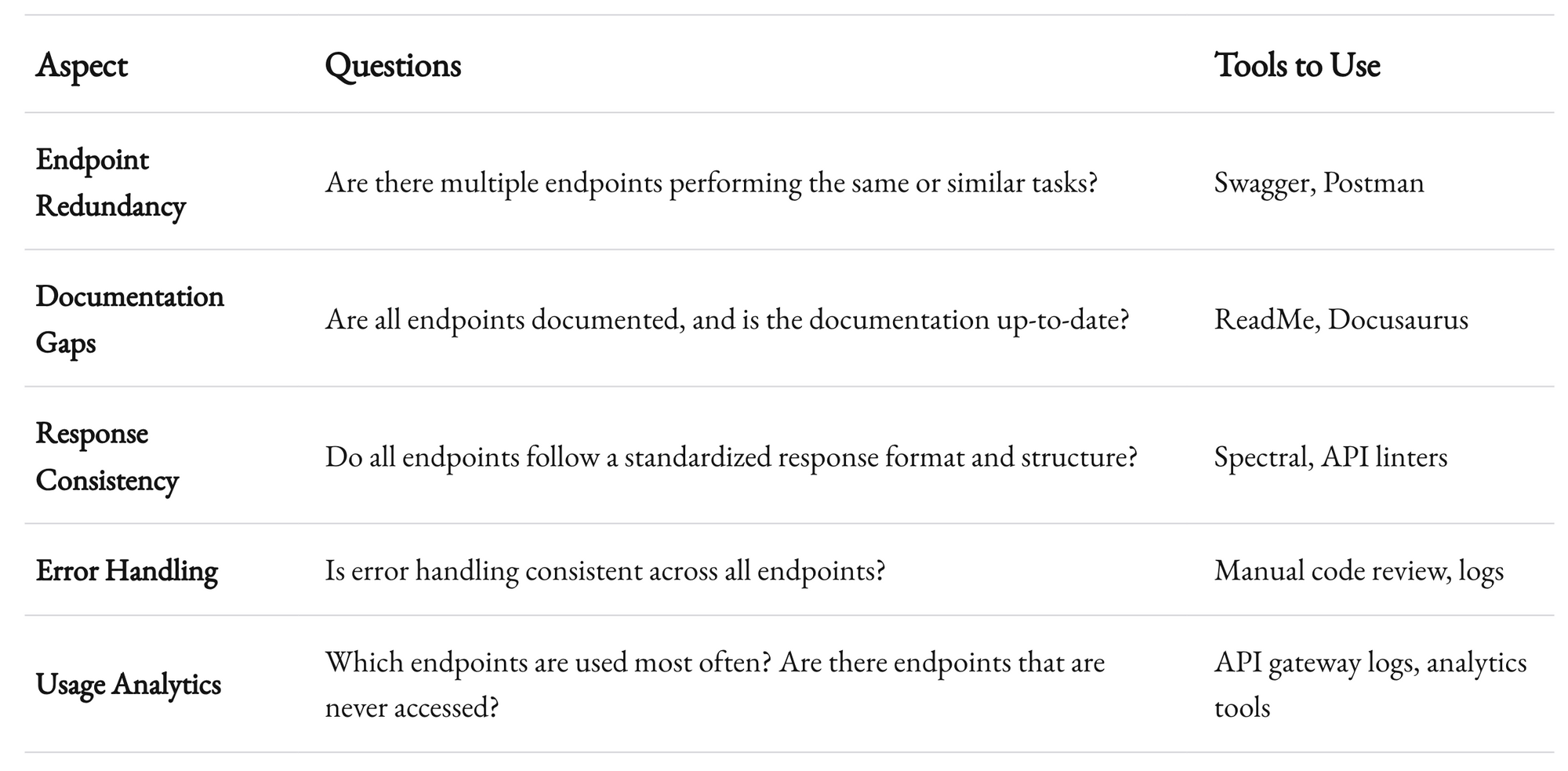
Step 2: Design for Consistency
Consistency is the antidote to chaos. A predictable API makes life easier for everyone—developers, integrators, and users.
Naming Conventions:
Stick to a clear, predictable naming convention. For example:
- Use Nouns for Resource Names:
Use nouns to represent resources (e.g.,/users
,/products
). This aligns with RESTful principles and makes endpoints intuitive. - Avoid Verbs in Endpoint Names:
Instead of/getUserData
, use HTTP methods to define actions (e.g.,GET /users
). This leverages the semantics of HTTP methods (GET, POST, PUT, DELETE) to convey actions, making the API more expressive and standardized. - Keep It Lowercase and Use Hyphens for Separation:
Use lowercase letters and hyphens to separate words (e.g.,/user-profiles
, not/UserProfiles
). This improves readability and consistency across different endpoints.

Standardize Response Formats:
Define a universal response structure. A common pattern is:
{
"data": {...},
"meta": {...},
"errors": [...]
}
- data: The actual response payload.
- meta: Metadata like pagination details.
- errors: List of error messages or codes, if any.
By standardizing this format, consumers know what to expect—every time. This consistency simplifies client-side parsing and error handling, reducing the cognitive load on developers.
Consistent Error Handling:
Define clear error codes and messages. For example:
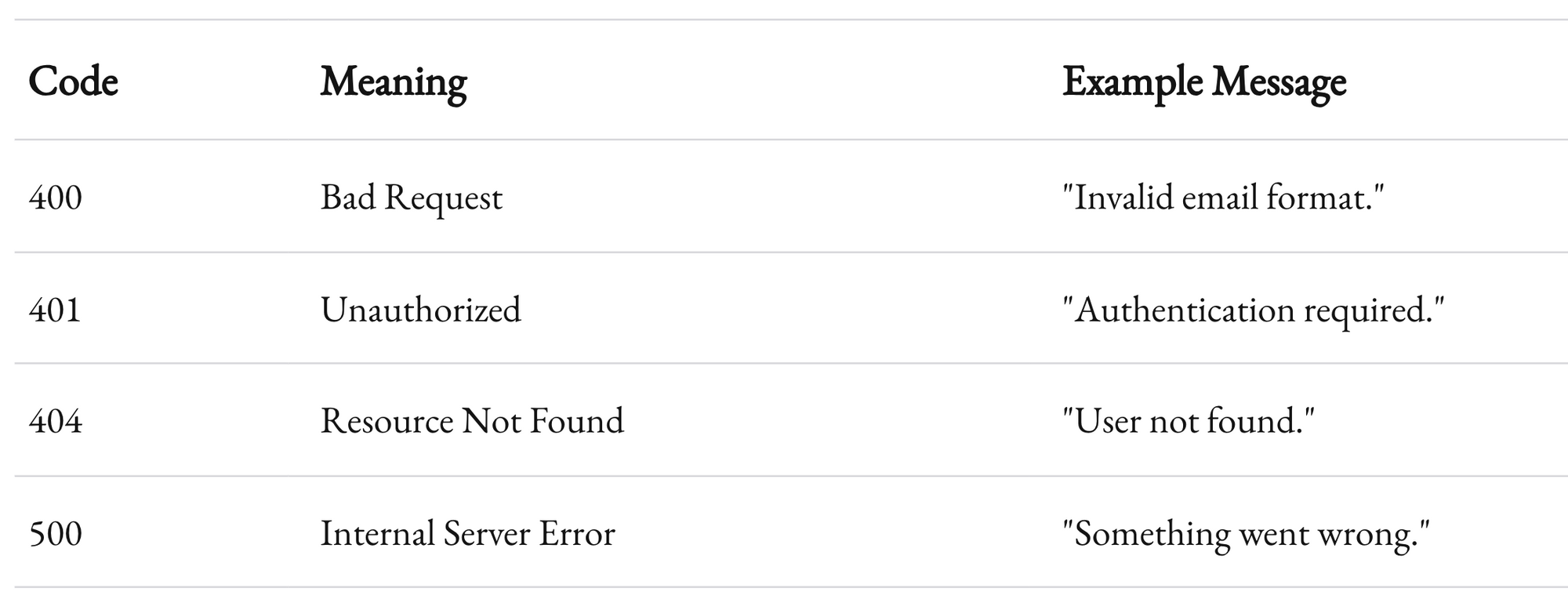
Document these errors so clients can programmatically handle them. Implementing standardized error responses ensures that developers can build robust and user-friendly applications by anticipating and managing potential issues effectively.
Additional Best Practices:
- Version Consistency: Ensure that all endpoints adhere to the same versioning strategy to prevent discrepancies and confusion.
- Pagination and Filtering: Implement consistent pagination, sorting, and filtering mechanisms across list endpoints to provide a uniform experience.
- Authentication and Authorization: Use a standardized approach for securing endpoints, such as OAuth 2.0 or JWT, to maintain uniformity in access control.
Step 3: Implement Versioning
Without proper versioning, you’ll eventually break someone’s integration. And that’s a recipe for angry emails.
Best Practices for Versioning:
Use URL-Based Versioning:
The simplest and most explicit approach. For example:
/v1/users
/v2/users
This makes it clear which version of the API is being used and allows multiple versions to coexist without conflict. URL-based versioning is straightforward to implement and easy for clients to understand and adopt.
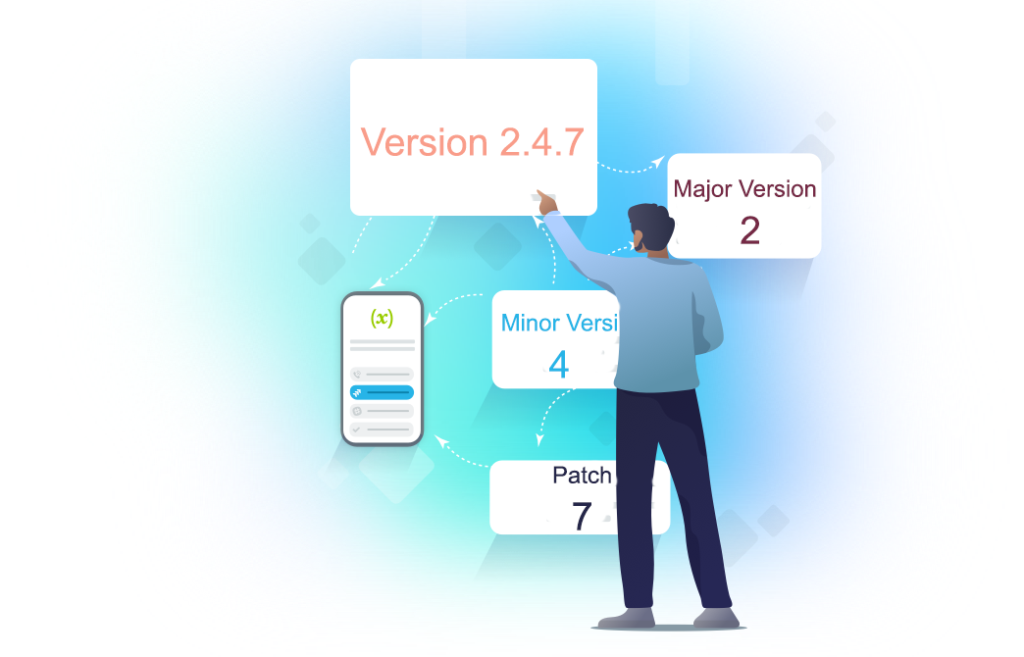
Deprecation Notices:
Communicate clearly when an older version is being retired. Provide migration guides to help users transition smoothly. For instance, announce deprecations well in advance, specify a timeline for retirement, and offer detailed instructions on how to upgrade to newer versions.
Semantic Versioning:
Adopt semantic versioning. Increment versions based on the type of changes:
- Major: Breaking changes.
- Minor: Backward-compatible feature additions.
- Patch: Backward-compatible bug fixes.
This approach provides a clear and predictable way to manage changes, helping clients understand the impact of updates and plan accordingly.
Real-World Example:
Stripe’s API is a gold standard for versioning. They include a Version
header in every API request, making it easy for clients to opt into changes at their own pace. This flexibility allows developers to adopt new features or changes without disrupting existing integrations, fostering a smooth and reliable user experience.
Additional Considerations:
- Backward Compatibility: Strive to maintain backward compatibility wherever possible to minimize disruptions for existing clients.
- Feature Flags: Use feature flags to toggle new features on or off, allowing gradual rollout and testing before full adoption.
- Documentation Updates: Ensure that all documentation is updated to reflect changes in each version, providing clear guidance on new features and deprecated elements.
Step 4: Refactor Gradually
Cleaning up an API doesn’t mean tearing everything down at once. Refactor incrementally to minimize risks and maintain system stability.
Start with Modularization:
Break down monolithic APIs into smaller, more manageable modules. For example, instead of:
GET /everything
Split functionality into:
GET /users
GET /orders
GET /products
This makes it easier to update and maintain individual components, reducing the complexity and improving the clarity of each module. Modularization also facilitates parallel development and easier testing, enhancing overall efficiency.
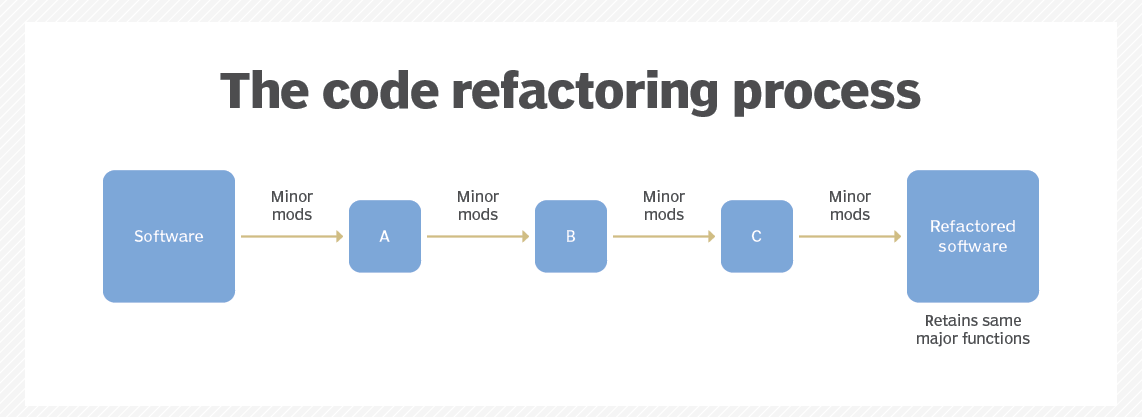
Implement Gateway Patterns:
Use an API gateway like Kong or AWS API Gateway. Gateways abstract the complexity of backend APIs, allowing you to:
- Route Requests: Direct traffic to the appropriate service based on the request path or other criteria.
- Apply Authentication and Rate Limiting: Enforce security and usage policies consistently across all endpoints.
- Transform Responses on the Fly: Modify responses to match standardized formats, ensuring consistency without altering backend services.
API gateways provide a centralized point for managing cross-cutting concerns, simplifying the overall architecture and enhancing scalability and security.
Additional Refactoring Strategies:
- Introduce Microservices: Transition from a monolithic architecture to microservices, allowing each service to handle a specific functionality. This promotes scalability, flexibility, and easier maintenance.
- Deprecate Unused Endpoints: Identify and remove endpoints that are no longer in use or redundant, reducing clutter and potential confusion.
- Optimize Database Interactions: Refactor APIs to optimize database queries and interactions, improving performance and reducing latency.
Pro Tip:
Implement feature toggles to manage the rollout of refactored components. This allows you to test changes in production without affecting all users immediately, providing a safety net during the transition.
Step 5: Automate Testing
Broken APIs erode trust. Automate testing to ensure changes don’t introduce new issues.
Types of Tests:
Unit Tests:
Test individual endpoints to ensure they behave as expected in isolation. For example, verify that GET /users
returns the correct user data and handles edge cases appropriately.
Integration Tests:
Validate that multiple services work together as expected. For instance, ensure that POST /orders
correctly interacts with the inventory and payment services to complete an order process.
Contract Tests:
Ensure APIs meet the agreed-upon contract with clients. Tools like Pact can help verify that the API adheres to the specified contracts, preventing unexpected changes that could break client integrations.
Load Tests:
Simulate real-world traffic to identify performance bottlenecks. Use tools like JMeter or Locust to stress-test your APIs under various load conditions, ensuring they can handle peak usage without degradation.
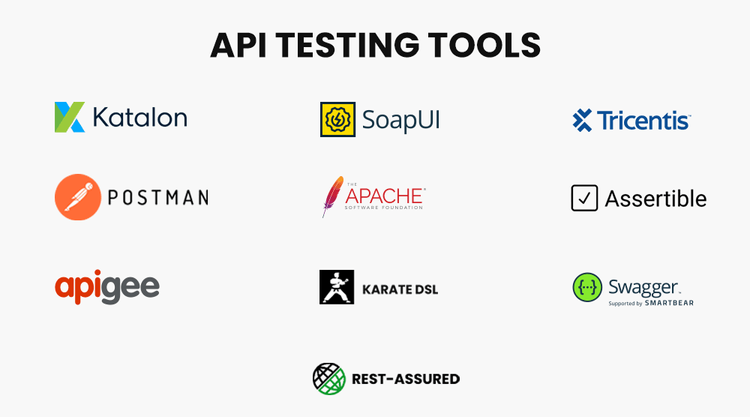
Tools to Use:
- Postman’s Newman:
Automate API testing by running Postman collections in CI/CD pipelines. Newman enables you to integrate API tests into your development workflow seamlessly. - JMeter:
Perform load testing to evaluate the performance and scalability of your APIs. JMeter can simulate heavy traffic and provide detailed reports on response times, throughput, and error rates. - Pact:
Implement contract testing to ensure that APIs adhere to defined contracts. Pact facilitates consumer-driven contract testing, promoting better collaboration between API providers and consumers.
Additional Best Practices:
- Continuous Integration (CI):
Integrate automated tests into your CI pipeline to ensure that every change is validated before deployment. This helps catch issues early and maintain a high level of code quality. - Test Coverage:
Strive for comprehensive test coverage, ensuring that all critical paths and edge cases are tested. Use coverage tools to identify and address gaps in your testing strategy. - Mock Services:
Use mock services to simulate dependencies during testing, enabling isolated and reliable test environments.
Pro Tip:
Implement a robust monitoring and alerting system to detect and respond to API issues in real-time. Tools like Datadog, New Relic, or Prometheus can provide insights into API performance and health, allowing proactive management of potential problems.
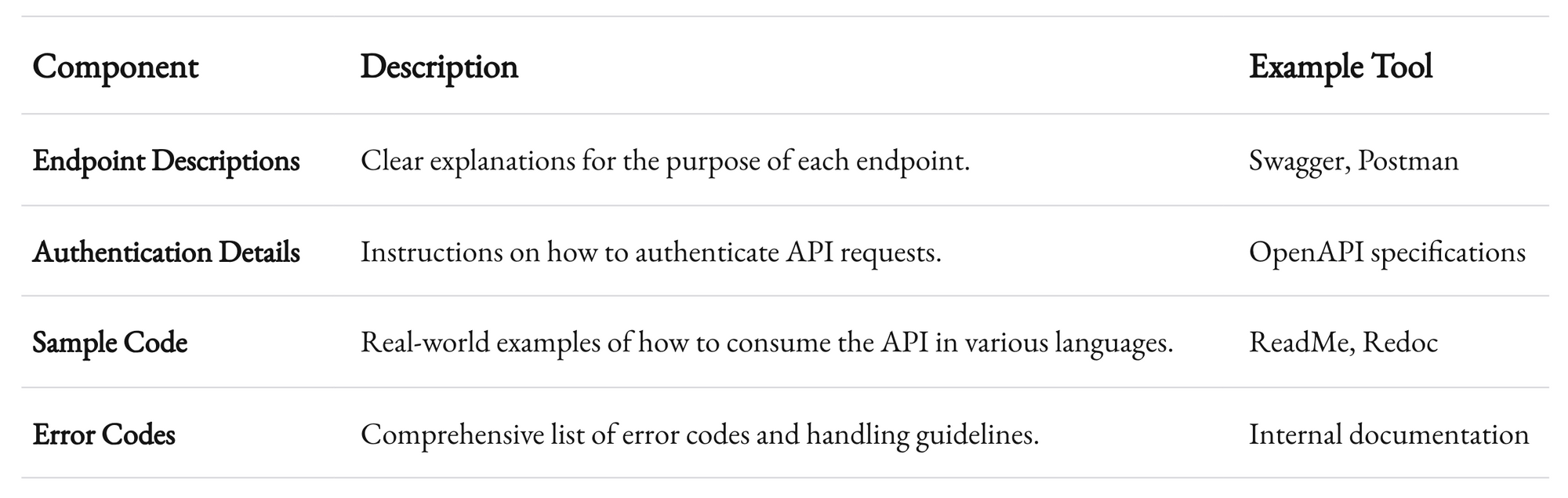
Step 6: Invest in Documentation
No matter how elegant your API is, poor documentation can render it useless. Documentation should be a first-class citizen in your development process.
What to Include:
Endpoint Descriptions:
Clearly explain each endpoint’s purpose, parameters, and expected outcomes. Provide context on how each endpoint fits into the broader system and its typical use cases.
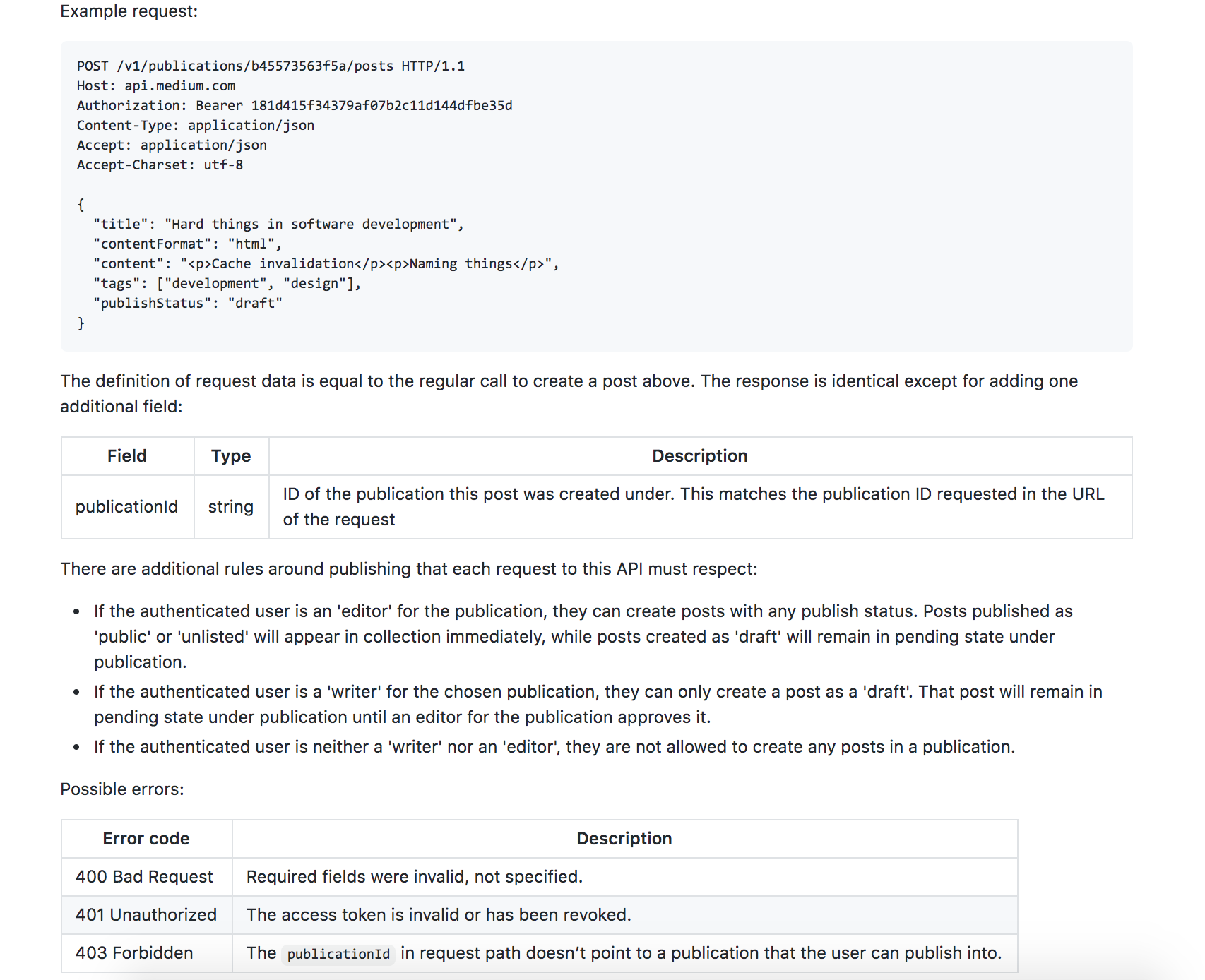
Sample Requests and Responses:
Provide real examples in multiple programming languages. This helps developers understand how to interact with the API and facilitates faster integration. For example:
# Sample GET request
curl -X GET "https://api.example.com/v1/users/123" \
-H "Authorization: Bearer YOUR_ACCESS_TOKEN"
{
"data": {
"id": "123",
"name": "John Doe",
"email": "[email protected]"
},
"meta": {},
"errors": []
}
Authentication Guidelines:
Detail how to authenticate and authorize requests. Explain the authentication mechanisms (e.g., OAuth 2.0, API keys, JWT), provide instructions on obtaining credentials, and outline best practices for secure usage.
Error Codes:
List all possible errors with explanations. Include error codes, messages, and guidance on how to resolve common issues. This empowers developers to handle errors gracefully and improve the overall user experience.
Tools to Simplify Documentation:
- Redoc:
Generate beautifully formatted API docs from OpenAPI specifications. Redoc offers customizable themes and interactive features that enhance the readability and usability of your documentation. - ReadMe:
Create interactive documentation platforms that allow users to test APIs directly from the documentation. ReadMe supports dynamic content and real-time updates, making it easier to maintain accurate and up-to-date docs. - Docusaurus:
Ideal for developer-focused content, Docusaurus enables you to build, deploy, and maintain documentation websites with ease. It supports versioning, search functionality, and integrations with various tools to enhance the documentation experience.
Additional Documentation Best Practices:
- Interactive Documentation:
Enable interactive elements like try-it-out features, code snippets, and live examples to engage developers and facilitate hands-on learning. - Search Functionality:
Implement robust search capabilities to help users quickly find the information they need, improving the overall usability of your documentation. - Versioned Documentation:
Maintain separate documentation for different API versions, ensuring that users can reference the correct information based on the version they are using.
Pro Tip:
Encourage feedback on your documentation to identify areas for improvement. Implement mechanisms for users to report issues, suggest enhancements, and ask questions, fostering a collaborative and responsive documentation environment.

The Business Case for Clean APIs
Clean APIs aren’t just a technical asset—they’re a business differentiator. They:
Improve Developer Experience:
Happier developers mean faster integration and fewer support tickets. A well-designed API with clear documentation and consistent behavior reduces the learning curve and minimizes the time developers spend troubleshooting issues, leading to increased productivity and satisfaction.
Enhance Scalability:
Clean, modular APIs handle growth better. As your user base and feature set expand, a well-structured API can scale seamlessly, accommodating increased traffic and new functionalities without compromising performance or reliability.
Boost Customer Retention:
Reliable APIs foster trust and loyalty. When customers can depend on your APIs for consistent and accurate results, they are more likely to continue using your services and recommend them to others, driving long-term growth and success.
Additional Business Benefits:
- Competitive Advantage:
Offering a superior API experience can set your product apart from competitors, attracting more developers and partners to your ecosystem. - Reduced Costs:
Minimizing technical debt and maintenance overhead through clean APIs can lead to significant cost savings over time, allowing you to allocate resources more effectively. - Faster Time-to-Market:
Streamlined APIs enable quicker development and deployment of new features, helping you respond to market demands and seize opportunities promptly.
Recap
API cleanup isn’t a one-time project. It’s an ongoing commitment to quality, consistency, and usability. By adopting these strategies, you’ll not only simplify your architecture but also build a stronger foundation for future growth.
At 1985, we’ve seen firsthand how clean APIs can transform projects. Whether you’re tackling a tangled legacy system or building fresh, our team is here to help. Because at the end of the day, no one likes spaghetti.
Ready to Clean Up Your API?
Contact us today to learn how our expertise can help you streamline your API architecture, enhance developer productivity, and scale your systems efficiently.