Advanced Android Development: Jetpack Compose UI
From state management to seamless animations, learn how Jetpack Compose transforms Android UI development.
Building Android apps has never been simple. Even with years of experience, every project brings its own puzzles. Jetpack Compose is Google’s answer to simplifying UI development. But, is it really the game-changer it promises to be? Let’s dive deep into the nuances and discover what makes Jetpack Compose a vital tool for advanced Android development.
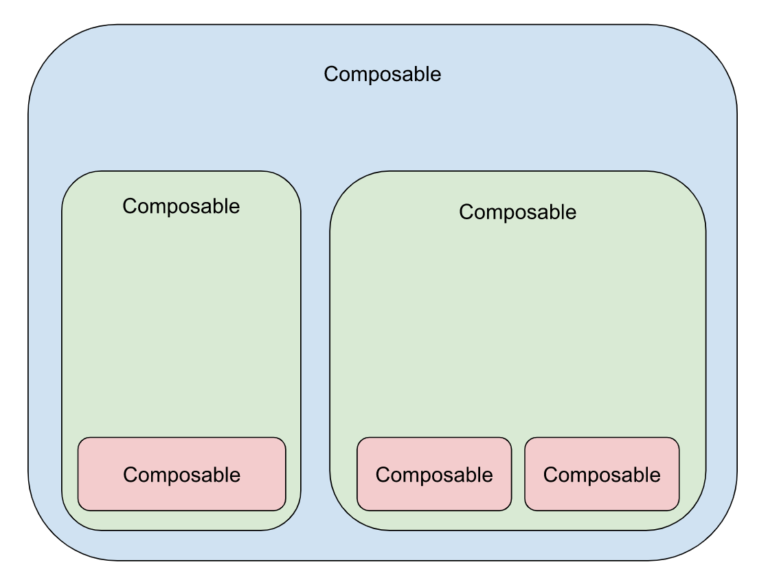
Why Jetpack Compose?
The question isn’t why. It’s why now. Android’s traditional UI toolkit, XML-based layouts, has been around for over a decade. While robust, it’s not without flaws—verbosity, limited flexibility, and the occasional UI-thread nightmare. Enter Jetpack Compose: a modern, declarative UI toolkit that addresses these pain points.
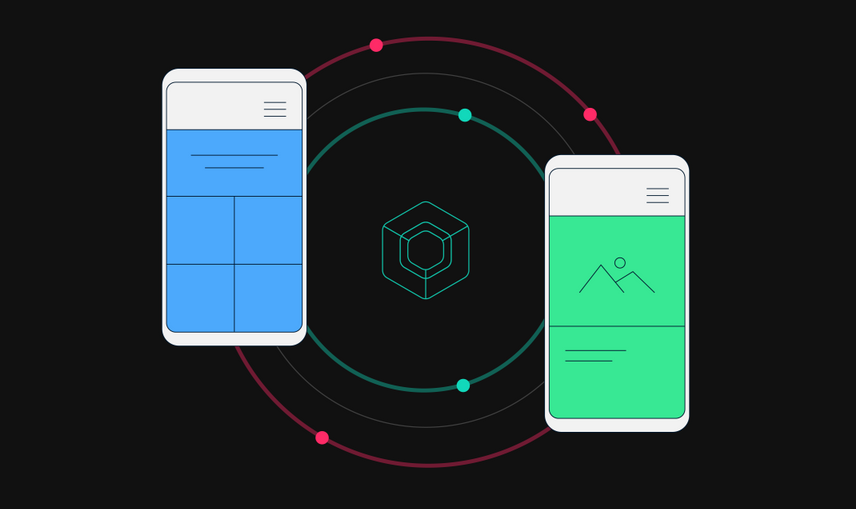
A Paradigm Shift
Jetpack Compose follows a declarative approach, unlike the imperative style of XML. You tell the framework what the UI should look like, not how to draw it. This shift is significant.
Take this simple example:
// Jetpack Compose Button
Button(onClick = { /* Handle click */ }) {
Text("Click Me")
}
Compare that with the XML + Java/ Kotlin equivalent:
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:onClick="onClickHandler"
android:text="Click Me" />
fun onClickHandler(view: View) {
// Handle click
}
The reduction in boilerplate is clear. And for a seasoned developer, that’s just the tip of the iceberg.
Composables: The Building Blocks
Every UI component in Jetpack Compose is a composable function. Want a list? Use LazyColumn
. Need a custom button? Write your own composable. These reusable and modular blocks encourage a clean, component-driven architecture—something modern apps can’t do without.
Features That Shine
Beyond the basics, Jetpack Compose brings features that make it indispensable for advanced projects.
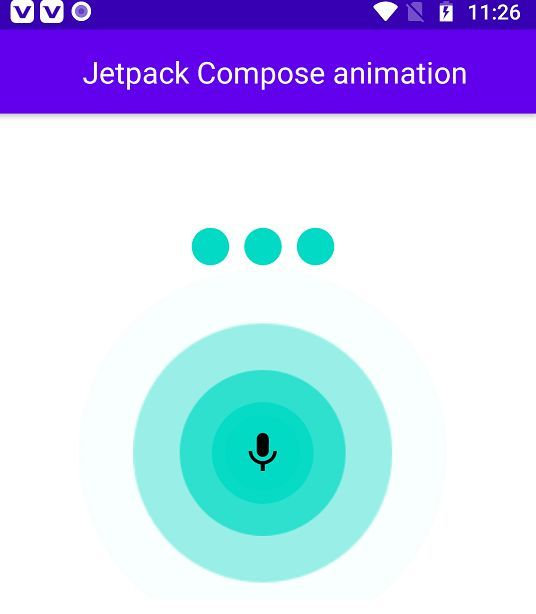
Real-Time Previews
No more waiting for the emulator to boot. With Android Studio’s Preview, you can instantly see changes. Modify your @Composable
, hit save, and voila—there’s your updated UI.
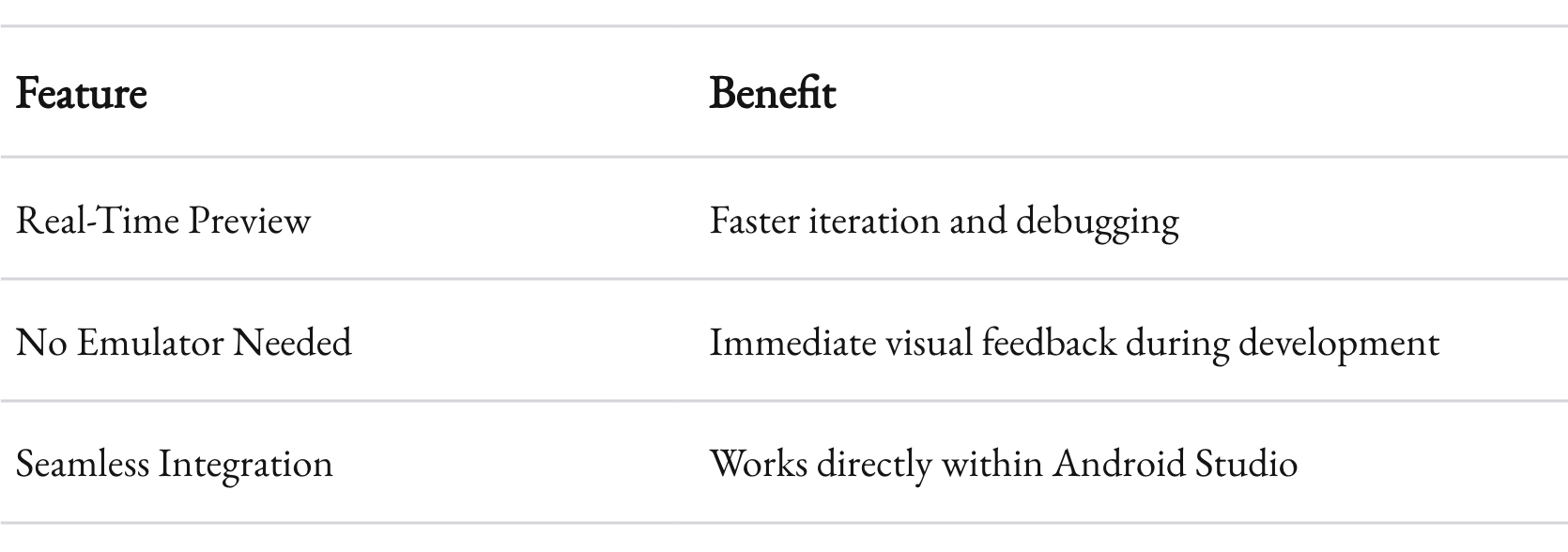
This isn’t just about convenience. It’s about speed and accuracy in iteration. You spend less time switching contexts and more time building.
State Management Done Right
State management in traditional Android can be tricky. Jetpack Compose, however, bakes state directly into its design. Using remember
and mutableStateOf
, you can manage UI state elegantly.
var count by remember { mutableStateOf(0) }
Button(onClick = { count++ }) {
Text("Count: $count")
}
Simple? Yes. Powerful? Even more so. Combine this with tools like Flow
or LiveData
, and you have a reactive, state-driven UI without the headache.
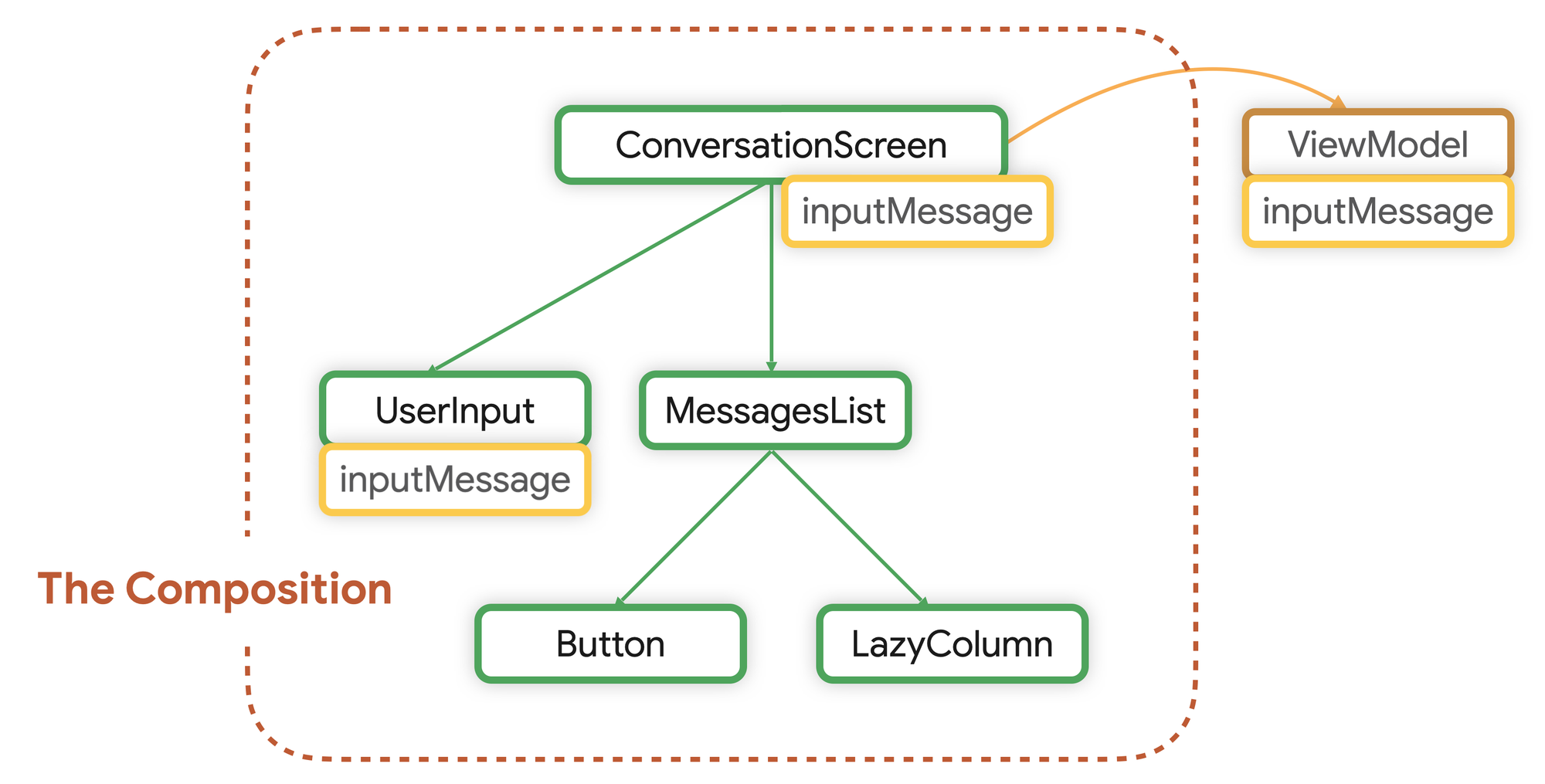
Animations: Simplified
Creating animations used to mean wrestling with ObjectAnimator
or XML. Compose’s declarative approach simplifies this.
Want to fade in a component? Here’s how:
val alpha by animateFloatAsState(targetValue = if (isVisible) 1f else 0f)
Box(modifier = Modifier.alpha(alpha)) {
Text("Hello, Compose!")
}
Advanced developers will appreciate how Compose handles complex motion seamlessly. Nested animations? Sequential transitions? Compose makes them intuitive.
Advanced Use Cases
Now that we’ve covered what makes Compose great, let’s explore how it handles advanced scenarios. Real-world apps are rarely about just buttons and lists.
Theming and Customization
Compose’s theming system is built around MaterialTheme
. But it’s not rigid. You can extend or replace it entirely to fit your brand’s identity.
Here’s how you might set up a custom typography:
val CustomTypography = Typography(
h1 = TextStyle(
fontFamily = FontFamily.Serif,
fontWeight = FontWeight.Bold,
fontSize = 30.sp
),
body1 = TextStyle(
fontFamily = FontFamily.Default,
fontSize = 16.sp
)
)
MaterialTheme(typography = CustomTypography) {
// Your composables
}
This level of control ensures your app’s look and feel remains consistent and unique.
Dynamic Lists
Dynamic lists are at the heart of most modern apps. Jetpack Compose’s LazyColumn
is a powerhouse. Need to load images dynamically? Use Coil
or Glide
within composables.
LazyColumn {
items(imageUrls) { url ->
Image(
painter = rememberImagePainter(data = url),
contentDescription = null
)
}
}
Testing: A First-Class Citizen
UI tests in Jetpack Compose are straightforward. With Compose Test, you can simulate user actions and verify behavior. This is a leap forward for teams focused on maintaining quality.
@Test
fun testButtonClick() {
composeTestRule.setContent {
MyComposable()
}
composeTestRule.onNodeWithText("Click Me").performClick()
composeTestRule.onNodeWithText("Count: 1").assertExists()
}
When your team can confidently test complex UI interactions, development speed and reliability improve dramatically.
Challenges to Watch Out For
No tool is perfect. Jetpack Compose, despite its brilliance, comes with a few caveats.
Performance Overheads
While Compose is optimized, misuse can lead to performance bottlenecks. Deep recompositions or unnecessary recomposables can slow things down. Profiling your app’s performance is crucial.
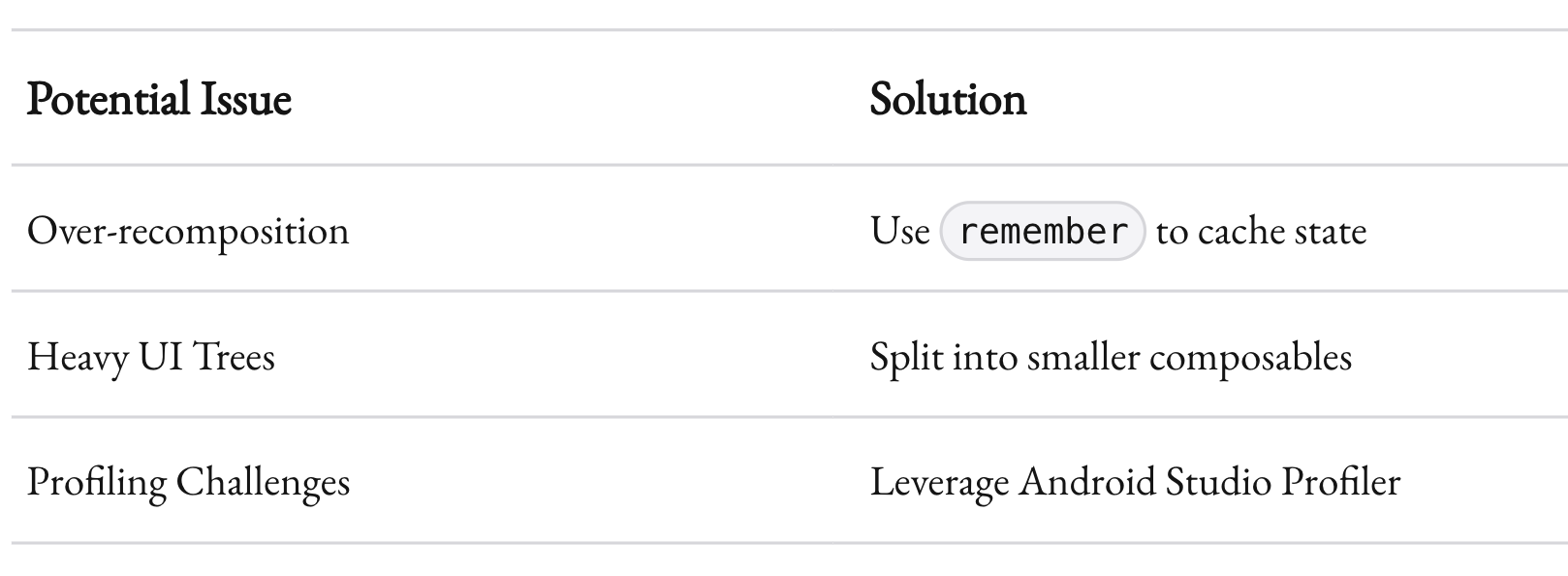
Learning Curve
Declarative paradigms are a mindset shift. For teams used to XML, adapting to Compose can take time. Proper training and incremental adoption are key.
Integration with Legacy Code
Most projects aren’t greenfield. Migrating an existing app to Compose requires careful planning, especially when bridging XML and Compose components.
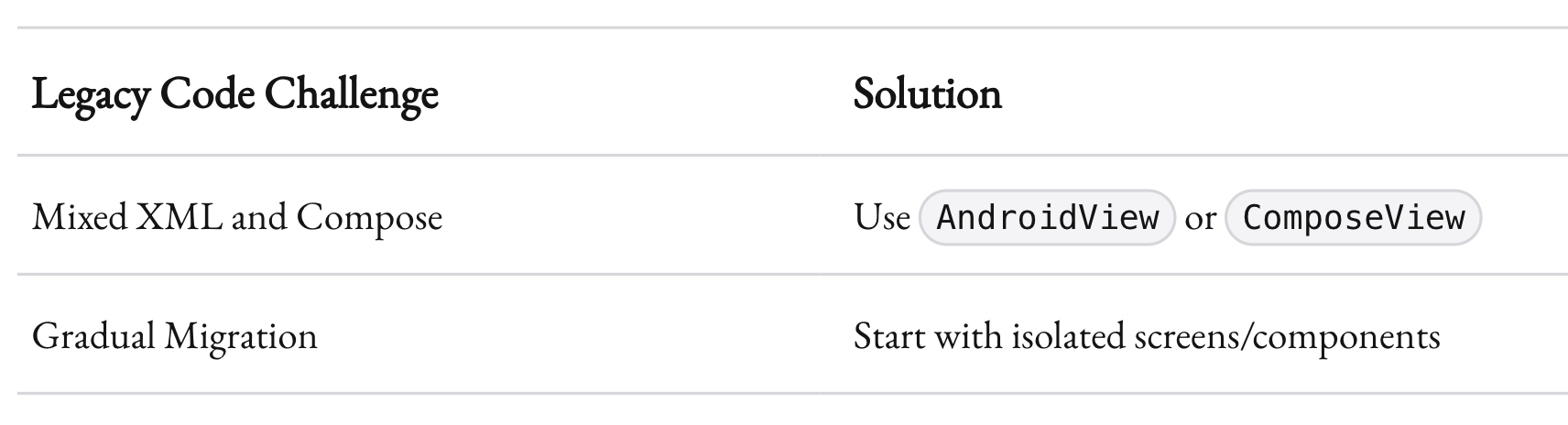
Jetpack Compose vs. Flutter
A common question: How does Compose stack up against Flutter? Both frameworks emphasize declarative UIs. However, Compose integrates seamlessly with the Android ecosystem. For teams already invested in Kotlin and Android, it’s the natural choice.
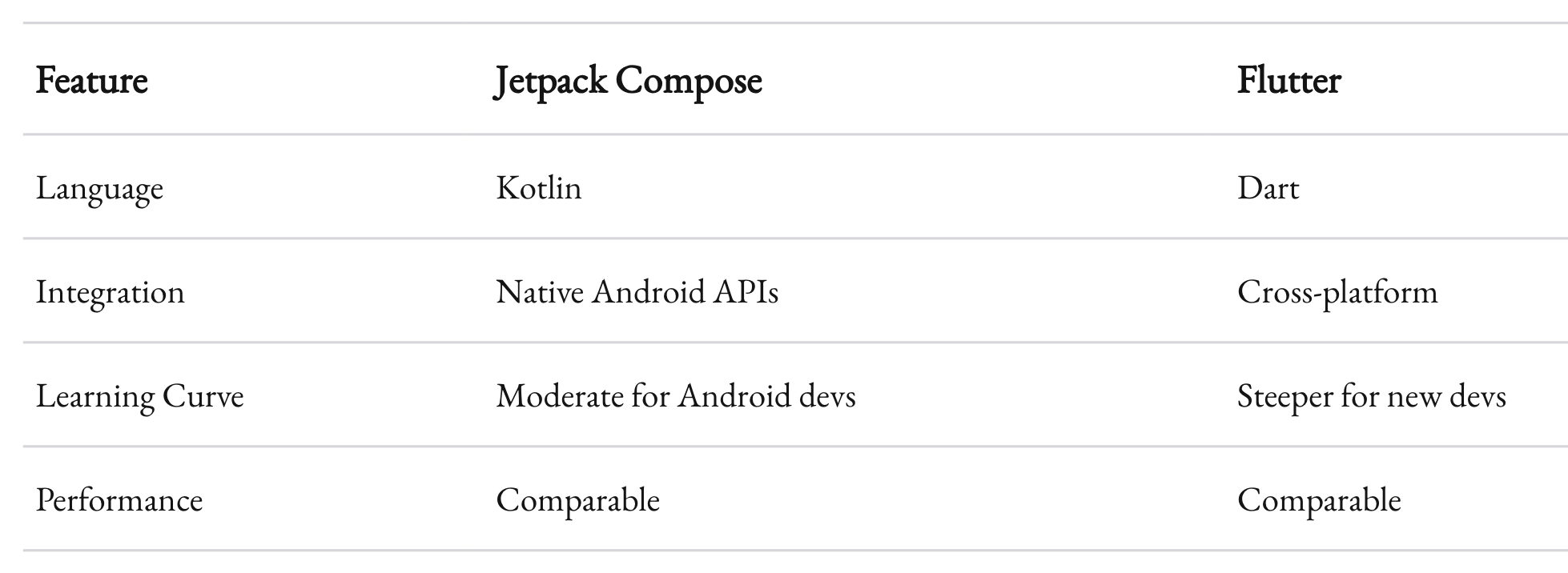
Each has its place. But if your focus is Android, Compose offers fewer compromises.
Real-World Success Stories
Several companies have already embraced Compose with impressive results. For instance, Airbnb switched to Compose for its search interface, citing faster development cycles and fewer bugs. According to a case study published by Google, developers saved 30% on implementation time.
Similarly, Netflix experimented with Compose for dynamic UI updates in its app—an effort that reduced memory consumption by 15%.
Future-Proof Your Android Development
Jetpack Compose isn’t just a trend. It’s a glimpse into the future of UI development on Android. By adopting it, you’re not just modernizing your apps. You’re also simplifying maintenance, improving testability, and enabling faster iterations.
As someone who runs an outsourced software development company, I’ve seen firsthand how Compose accelerates projects. Clients love faster delivery. Developers enjoy fewer headaches. And users? They get sleek, responsive UIs.
The question isn’t whether to adopt Jetpack Compose. It’s when. And if you’re building for Android, the answer is yesterday.